#include
#include
#include
#include
#define MAX 10000
char * multiply(char [],char[]);
int main(){
char a[MAX];
char b[MAX];
char *c;
int la,lb;
int i;
printf("Enter the first number : ");
scanf("%s",a);
printf("Enter the second number : ");
scanf("%s",b);
printf("Multiplication of two numbers : ");
c = multiply(a,b);
printf("%s",c);
return 0;
}
char * multiply(char a[],char b[]){
static char mul[MAX];
char c[MAX];
char temp[MAX];
int la,lb;
int i,j,k=0,x=0,y;
long int r=0;
long sum = 0;
la=strlen(a)-1;
lb=strlen(b)-1;
for(i=0;i<=la;i++){
a[i] = a[i] - 48;
}
for(i=0;i<=lb;i++){
b[i] = b[i] - 48;
}
for(i=lb;i>=0;i--){
r=0;
for(j=la;j>=0;j--){
temp[k++] = (b[i]*a[j] + r)%10;
r = (b[i]*a[j]+r)/10;
}
temp[k++] = r;
x++;
for(y = 0;y<x;y++){
temp[k++] = 0;
}
}
k=0;
r=0;
for(i=0;i<la+lb+2;i++){
sum =0;
y=0;
for(j=1;j<=lb+1;j++){
if(i <= la+j){
sum = sum + temp[y+i];
}
y += j + la + 1;
}
c[k++] = (sum+r) %10;
r = (sum+r)/10;
}
c[k] = r;
j=0;
for(i=k-1;i>=0;i--){
mul[j++]=c[i] + 48;
}
mul[j]='\0';
return m
multiplications Table of large numbers in C
multiplications Table of large numbers in C | large numbers Calculations in C | large numbers Programs in C | Long Integers In C
Labels:
C Assignments
C program to find power of a large number
C program to find power of a large number | Power of Large Numbers in C | Find Power Of Numbers in C Language
#include
#include
#define MAX 10000
char * multiply(char [],char[]);
int main(){
char a[MAX];
char *c;
int i,n;
printf("Enter the base number: ");
scanf("%s",a);
printf("Enter the power: ");
scanf("%d",&n);
printf("Power of the %s^%d: ",a,n);
c = multiply(a,"1");
for(i=0;i<n-1;i++)
c = multiply(a,c);
while(*c)
if(*c =='0')
c++;
else
break;
printf("%s",c);
return 0;
}
char * multiply(char num1[],char num2[]){
static char mul[MAX];
char a[MAX];
char b[MAX];
char c[MAX];
char temp[MAX];
int la,lb;
int i=0,j,k=0,x=0,y;
long int r=0;
long sum = 0;
while(num1[i]){
a[i] = num1[i];
i++;
}
a[i]= '\0';
i=0;
while(num2[i]){
b[i] = num2[i];
i++;
}
b[i]= '\0';
la=strlen(a)-1;
lb=strlen(b)-1;
for(i=0;i<=la;i++){
a[i] = a[i] - 48;
}
for(i=0;i<=lb;i++){
b[i] = b[i] - 48;
}
for(i=lb;i>=0;i--){
r=0;
for(j=la;j>=0;j--){
temp[k++] = (b[i]*a[j] + r)%10;
r = (b[i]*a[j]+r)/10;
}
temp[k++] = r;
x++;
for(y = 0;y<x;y++){
temp[k++] = 0;
}
}
k=0;
r=0;
for(i=0;i<la+lb+2;i++){
sum =0;
y=0;
for(j=1;j<=lb+1;j++){
if(i <= la+j){
sum = sum + temp[y+i];
}
y += j + la + 1;
}
c[k++] = (sum+r) %10;
r = (sum+r)/10;
}
c[k] = r;
j=0;
for(i=k-1;i>=0;i--){
mul[j++]=c[i] + 48;
}
mul[j]='\0';
return mul;
}
Related Links :
C Program to remove duplicate elements from given array
C Program to remove duplicate elements from given array | deleting Duplicate Elements from array
#include
int main(){
int arr[50];
int *p;
int i,j,k,size,n;
printf("\nEnter size of the array: ");
scanf("%d",&n);
printf("\nEnter %d elements into the array: ",n);
for(i=0;i<n;i++)
scanf("%d",&arr[i]);
size=n;
p=arr;
for(i=0;i<size;i++){
for(j=0;j<size;j++){
if(i==j){
continue;
}
else if(*(p+i)==*(p+j)){
k=j;
size--;
while(k < size){
*(p+k)=*(p+k+1);
k++;
}
j=0;
}
}
}
printf("\nThe array after removing duplicates is: ");
for(i=0;i < size;i++){
printf(" %d",arr[i]);
}
return 0;
}
Related Links :
Labels:
C Assignments
Find 2nd Largest Number From Array in C || C program to find the second largest element in an array
Find 2nd Largest Number From Array in C || C program to find the second largest element in an array
#include
int main(){
int a[50],size,i,j=0,big,secondbig;
printf("Enter the size of the array: ");
scanf("%d",&size);
printf("Enter %d elements in to the array: ", size);
for(i=0;i<size;i++)
scanf("%d",&a[i]);
big=a[0];
for(i=1;i<size;i++){
if(big<a[i]){
big=a[i];
j = i;
}
}
secondbig=a[size-j-1];
for(i=1;i<size;i++){
if(secondbig <a[i] && j != i)
secondbig =a[i];
}
printf("Second biggest: %d", secondbig);
return 0;
}
Related Links :
Labels:
C Assignments
Program to Add or subtract two complex numbers
Program to Add or subtract two complex numbers
#include
int main(){
int a,b,c,d,x,y;
printf("\nEnter the first complex number:");
scanf("%d%d",&a,&b);
printf("\nEnter the second complex number:");
scanf("%d%d",&c,&d);
if(b<0)
printf("%d-i\n",a-b);
else
printf("d+i\n",a+b);
if(d<0)
printf("d-i\n",c-d);
else
printf("%d+i\n",c+d);
printf("\nADDITION ");
x=a+c;
y=b+d;
if(y>0)
printf("%d-i%d",x,-y);
else
printf("%d+i%d",x,+y);
printf("\n\nSUBTRACTION ");
x=a-c;
y=b-d;
if(y<0)
printf("%d-i%d",x,-y);
else
printf("%d+i%d",x,+y);
return 0;
}
Related Links :
Labels:
C Assignments
C program for cleardevice | cleardevice attached in C
C program for cleardevice | cleardevice attached in C
#include
#include
main()
{
int gd = DETECT, gm;
initgraph(&gd, &gm, "C:\\TC\\BGI");
outtext("Press any key to clear the screen.");
getch();
cleardevice();
outtext("Press any key to exit...");
getch();
closegraph();
return 0;
}
Related Links :
draw a circle in C | Cirlce Graphic in C
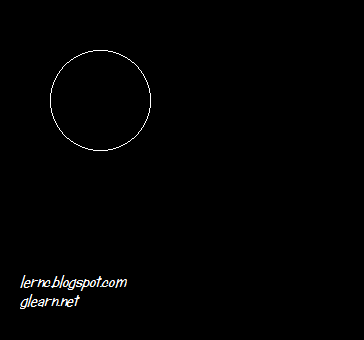
draw a circle in C
#include
#include
main()
{
int gd = DETECT, gm;
initgraph(&gd, &gm, "C:\\TC\\BGI");
circle(100, 100, 50);
getch();
closegraph();
return 0;
}
Related Links :
Labels:
Graphics In C
arc graphics function in c | Drawing Arc in C
C Program to change system date | SetDate Function in C | Change System Clock Time From C
C Program to change system date | SetDate Function in C | Change System Clock Time From C
#include
#include
#include
main()
{
/*Program to change System Date*/
struct date d;
printf("Enter the new date ( day, month and year ) as integers ");
scanf("%d%d%d",&d.da_day,&d.da_mon,&d.da_year);
setdate(&d);
printf("Now Current system date is %d/%d/%d\n",d.da_day,d.da_mon,d.da_year);
getch();
return 0;
}
Related Links :
gettime() Function in C
gettime() Function in C
Returns System Time.
Returns System Time.
#include
#include
main()
{
struct time t;
gettime(&t);
printf("Current system time is %d : %d : %d\n",t.ti_hour,t.ti_min,t.ti_sec);
return 0;
}
Related Links :
Labels:
gettime() Function in C
Getdate Function in C
Getdate Function in C
#include
#include
main()
{
struct date d;
getdate(&d);
printf("Current system date is %d/%d/%d\n",d.da_day,d.da_mon,d.da_year);
return 0;
}
Related Links :
Labels:
Getdate Function in C
delay function in c
To use delay function in your program you should include the dos.h header file.
#include
#include
main()
{
printf("This c program will exit in 10 seconds.\n");
delay(10000);
return 0;
}
Related Links :
using chdir in c, chdir function in c,
chdir Example program in C, chdir Demo in C, program using chdir function in C
#include
#include
#include
#include
const char * const path = "d:/popsys/test";
const char * const file = "lernc.txt";
int main () {
printf ("Changing directory to <%s>\n", path);
if (chdir (path) == -1) {
printf ("chdir failed - %s\n", strerror (errno));
}else {
printf ("chdir done !!!\n");
printf ("directory content of '%s'\n\n", path);
system ("ls -l");
printf ("\n");
printf ("checking the content of '%s'\n", file);
system ("cat lernc.txt");
}
return 0;
}
Related Links :
fopen example in c, use of fopen function in C, C File handling
fopen example in c, use of fopen function in C, C File handling
#include
void main()
{
FILE *fopen(), *fp;
int c ;
fp = fopen( "lernc.com", "r" ); /*open file for reading */
c = getc( fp ) ;
while ( c != EOF )
{
putchar( c );
c = getc ( fp );
}
fclose( fp );
}
Related Links :
c Program to count characters in given file, Count characters
c Program to count characters in given file, Count characters in from file, number of characters in given file
#include
void main()
{
FILE *fopen(), *fp;
int c , nc, nlines;
char filename[40] ;
nlines = 0 ;
nc = 0;
printf("Enter file name: ");
gets( filename );
fp = fopen( filename, "r" );
if ( fp == NULL )
{
printf("Cannot open %s for reading \n", filename );
exit(1); /* terminate program */
}
c = getc( fp ) ;
while ( c != EOF )
{
if ( c == '\n' )
nlines++ ;
nc++ ;
c = getc ( fp );
}
fclose( fp );
if ( nc != 0 )
{
printf("There are %d characters in %s \n", nc, filename );
printf("There are %d lines \n", nlines );
}
else
{
printf("File: %s is empty \n", filename );
}
}
Related Links :
c program to count number of lines in a file
c program to count number of lines in a file, Number of lines in given text file
#include
void main()
{
FILE *fopen(), *fp;
int c , nc, nlines;
char filename[40] ;
nlines = 0 ;
nc = 0;
printf("Enter the file name: ");
gets( filename );
fp = fopen( filename, "r" );
if ( fp == NULL )
{
printf("Sorry Cannot open %s for reading \n", filename );
exit(1); /* End program */
}
c = getc( fp ) ;
while ( c != EOF )
{
if ( c == '\n' )
nlines++ ;
nc++ ;
c = getc ( fp );
}
fclose( fp );
if ( nc != 0 )
{
printf("There are %d characters in %s \n", nc, filename );
printf("There are %d lines \n", nlines );
}
else
{
printf("Ohh.h....File: %s is empty \n", filename );
}
}
Related Links :
c program to compare two files without using predefined Function
c program to compare two files without using predefined Function
#include
void main()
{
FILE *fp1, *fp2, *fopen();
int ca, cb;
char fname1[40], fname2[40] ;
printf("Enter name of first file :") ;
gets(fname1);
printf("Enter name of second file:");
gets(fname2);
fp1 = fopen( fname1, "r" ); /* open file1 for reading */
fp2 = fopen( fname2, "r" ) ; /* open file 2 for reading */
if ( fp1 == NULL ) /* check does file exist etc */
{
printf("Cannot open %s for reading \n", fname1 );
exit(1); /* terminate program */
}
else if ( fp2 == NULL )
{
printf("Cannot open %s for reading \n", fname2 );
exit(1); /* terminate program */
}
else /* both files opened successfully */
{
ca = getc( fp1 ) ;
cb = getc( fp2 ) ;
while ( ca != EOF && cb != EOF && ca == cb )
{
ca = getc( fp1 ) ;
cb = getc( fp2 ) ;
}
if ( ca == cb )
printf("Files are identical \n");
else if ( ca != cb )
printf("Files differ \n" );
fclose ( fp1 );
fclose ( fp2 );
}
}
Related Links :
Program to Move car in C
Program to Move car in C,C program to move a car, car running program in C, car game in C
#include
#include
#include
main()
{
int i, j = 0, gd = DETECT, gm;
initgraph(&gd,&gm,"C:\\TC\\BGI");
settextstyle(DEFAULT_FONT,HORIZ_DIR,2);
outtextxy(25,240,"Press any key to view the moving car");
getch();
setviewport(0,0,639,440,1);
for( i = 0 ; i <= 420 ; i = i + 10, j++ )
{
rectangle(50+i,275,150+i,400);
rectangle(150+i,350,200+i,400);
circle(75+i,410,10);
circle(175+i,410,10);
setcolor(j);
delay(100);
if( i == 420 )
break;
clearviewport();
}
getch();
closegraph();
return 0;
}
Related Links :
Labels:
Program to Move car in C
Creating captcha in C, captcha program in c
Creating captcha in C, captcha program in c, Captcha in C, C Language to show captcha
#include
#include
#include
main()
{
int i = 0, key, num, midx, gd = DETECT, gm;
char a[10];
initgraph(&gd,&gm,"C:\\TC\\BGI");
midx = getmaxx()/2;
settextstyle(SCRIPT_FONT,HORIZ_DIR,5);
settextjustify(CENTER_TEXT,CENTER_TEXT);
setcolor(GREEN);
outtextxy(midx,20,"CAPTCHA");
settextstyle(SCRIPT_FONT,HORIZ_DIR,2);
outtextxy(midx,125,"Press any key to change the generated random code \"captcha\"");
outtextxy(midx,150,"Press escape key to exit...");
setcolor(WHITE);
setviewport(100,200,600,400,1);
setcolor(RED);
randomize();
while(1)
{
while(i<6)
{
num = random(3);
if ( num == 0 )
a[i] = 65 + random(26); /* 65 is the ASCII value of A */
else if ( num == 1)
a[i] = 97 + random(26); /* 97 is the ASCII value of a */
else
a[i] = 48 + random(10); /* 48 is the ASCII value of 0 */
i++;
}
a[i] = '\0';
outtextxy(210,100,a);
key = getch();
if( key == 27 ) /* escape key*/
exit(0);
clearviewport();
i = 0;
}
}
Related Links :
traffic light program in c, traffic light simulation in C
traffic light program in c, traffic light simulation in C
#include
#include
#include
#include
main()
{
int gd = DETECT, gm, midx, midy;
initgraph(&gd, &gm, "C:\\TC\\BGI");
midx = getmaxx()/2;
midy = getmaxy()/2;
setcolor(RED);
settextstyle(SCRIPT_FONT, HORIZ_DIR, 3);
settextjustify(CENTER_TEXT, CENTER_TEXT);
outtextxy(midx, midy-10, "Traffic Light Simulation");
outtextxy(midx, midy+10, "Press any key to start");
getch();
cleardevice();
setcolor(WHITE);
settextstyle(DEFAULT_FONT, HORIZ_DIR, 1);
rectangle(midx-30,midy-80,midx+30,midy+80);
circle(midx, midy-50, 22);
setfillstyle(SOLID_FILL,RED);
floodfill(midx, midy-50,WHITE);
setcolor(BLUE);
outtextxy(midx,midy-50,"STOP");
delay(2000);
graphdefaults();
cleardevice();
setcolor(WHITE);
rectangle(midx-30,midy-80,midx+30,midy+80);
circle(midx, midy, 20);
setfillstyle(SOLID_FILL,YELLOW);
floodfill(midx, midy,WHITE);
setcolor(BLUE);
outtextxy(midx-18,midy-3,"READY");
delay(2000);
cleardevice();
setcolor(WHITE);
rectangle(midx-30,midy-80,midx+30,midy+80);
circle(midx, midy+50, 22);
setfillstyle(SOLID_FILL,GREEN);
floodfill(midx, midy+50,WHITE);
setcolor(BLUE);
outtextxy(midx-7,midy+48,"GO");
setcolor(RED);
settextstyle(SCRIPT_FONT, HORIZ_DIR, 4);
outtextxy(midx-150, midy+100, "Press any key to exit...");
getch();
closegraph();
return 0;
}
Related Links :
Best fortran compiler for Windows 7
I think Best FORTRAN compiler for Windows 7 are follows :
http://www.gnu.org/software/gcc/
http://gcc.gnu.org/install/binaries.html
http://www.gnu.org/software/gcc/
http://gcc.gnu.org/install/binaries.html
Related Links :
Program to Calculating interest C
Program to Calculating interest C
double recursive(double base, int runs) {
static double m_rate = 1 + (10/100); /* Ten percent interest*/
double new_base = (base*m_rate);
if (runs != 0)
return recursive(new_base,runs-1);
else
return new_base;
}
int main() {
printf("%.2f",recursive(100,10));
return 0;
}
Related Links :
C Program 2D arrays with structs and pointers
C Program 2D arrays with structs and pointers
#include
#include
#define NUM_ROWS 20
#define NUM_COLS 20
#define WALL 0
#define FLOOR 1
#define PASS 1
#define NOT_PASS 0
typedef struct playerChar {
int HP;
} PlayerChar;
//General struct with pointers to every type of 'creature' that can be shown on map
typedef struct charPointers {
PlayerChar * pPointer;
} CharPointers;
int main (void) {
initscr();
noecho();
curs_set(0);
resize_term (NUM_ROWS, NUM_COLS);
int i, j;
int ** mapArray = (int **) malloc (NUM_ROWS * sizeof (int *));
int ** passableArray = (int **) malloc (NUM_ROWS * sizeof (int *));
int ** charLoc = (int **) malloc (NUM_ROWS * sizeof (int *));
for (i = 0; i < NUM_ROWS; i++) {
mapArray [i] = (int *) malloc (NUM_COLS * sizeof (int));
passableArray [i] = (int *) malloc (NUM_COLS * sizeof (int));
charLoc [i] = (int *) malloc (NUM_COLS * sizeof (CharPointers));
for (j = 0; j < NUM_COLS; j++) {
if (i == 0) {
mapArray [i][j] = WALL;
passableArray [i][j] = NOT_PASS;
}
else if (i == (NUM_ROWS - 1)) {
mapArray [i][j] = WALL;
passableArray [i][j] = NOT_PASS;
}
else if (j == 0) {
mapArray [i][j] = WALL;
passableArray [i][j] = NOT_PASS;
}
else if (j == (NUM_COLS - 1)) {
mapArray [i][j] = WALL;
passableArray [i][j] = NOT_PASS;
}
else {
mapArray [i][j] = FLOOR;
passableArray [i][j] = PASS;
}
charLoc[i][j].CharPointers.pPointer = NULL;
}
}
Related Links :
read an electric signal through sound card c++
read an electric signal through sound card
CWaveFile g_pWaveFile;
WAVEFORMATEX wfxInput;
ZeroMemory( &wfxInput, sizeof(wfxInput));
wfxInput.wFormatTag = WAVE_FORMAT_PCM;
wfxInput.nSamplesPerSec = 22050
wfxInput.wBitsPerSample = 8;
wfxInput.nChannels = 1;
wfxInput.nBlockAlign =
wfxInput.nChannels * (wfxInput.wBitsPerSample / 8);
wfxInput.nAvgBytesPerSec =
wfxInput.nBlockAlign * wfxInput.nSamplesPerSec;
g_pWaveFile = new CWaveFile;
if (FAILED(g_pWaveFile->Open("mywave.wav", &wfxInput,
WAVEFILE_WRITE)))
{
g_pWaveFile->Close();
}
=============================================
HRESULT RecordCapturedData()
{
HRESULT hr;
VOID* pbCaptureData = NULL;
DWORD dwCaptureLength;
VOID* pbCaptureData2 = NULL;
DWORD dwCaptureLength2;
VOID* pbPlayData = NULL;
UINT dwDataWrote;
DWORD dwReadPos;
LONG lLockSize;
if (NULL == g_pDSBCapture)
return S_FALSE;
if (NULL == g_pWaveFile)
return S_FALSE;
if (FAILED (hr = g_pDSBCapture->GetCurrentPosition(
NULL, &dwReadPos)))
return hr;
// Lock everything between the private cursor
// and the read cursor, allowing for wraparound.
lLockSize = dwReadPos - g_dwNextCaptureOffset;
if( lLockSize < 0 ) lLockSize += g_dwCaptureBufferSize;
if( lLockSize == 0 ) return S_FALSE;
if (FAILED(hr = g_pDSBCapture->Lock(
g_dwNextCaptureOffset, lLockSize,
&pbCaptureData, &dwCaptureLength,
&pbCaptureData2, &dwCaptureLength2, 0L)))
return hr;
// Write the data. This is done in two steps
// to account for wraparound.
if (FAILED( hr = g_pWaveFile->Write( dwCaptureLength,
(BYTE*)pbCaptureData, &dwDataWrote)))
return hr;
if (pbCaptureData2 != NULL)
{
if (FAILED(hr = g_pWaveFile->Write(
dwCaptureLength2, (BYTE*)pbCaptureData2,
&dwDataWrote)))
return hr;
}
// Unlock the capture buffer.
g_pDSBCapture->Unlock( pbCaptureData, dwCaptureLength,
pbCaptureData2, dwCaptureLength2 );
// Move the capture offset forward.
g_dwNextCaptureOffset += dwCaptureLength;
g_dwNextCaptureOffset %= g_dwCaptureBufferSize;
g_dwNextCaptureOffset += dwCaptureLength2;
g_dwNextCaptureOffset %= g_dwCaptureBufferSize;
return S_OK;
}
Related Links :
c program for arriving at a solution using bisection method
c program for arriving at a solution using bisection method
main()
{
float a,b,c,x1,x2,x,series;
double d;
printf("enter a,b,c and x1(pos) & x2(neg)");
scanf("%f%f%f%f%f", &a, &b, &c, &x1, &x2);
read:
x = (x1 + x2) / 2;
series = a * x * x + b * x + c;
d = fabs(series);
if (d > 0.0001)
{
if (x * x1 < 0)
x = x2;
else
x = x1;
goto read;
}
else
{
printf("ans=%f", x);
}
return 0;
}
Related Links :
Subscribe to:
Posts (Atom)
If you face any Problem in viewing code such as Incomplete "For Loops" or "Incorrect greater than or smaller" than equal to signs then please collect from My Web Site CLICK HERE
More Useful Topics... |
|
History Of C..
In the beginning was Charles Babbage and his Analytical Engine, a machine
he built in 1822 that could be programmed to carry out different computations.
Move forward more than 100 years, where the U.S. government in
1942 used concepts from Babbage’s engine to create the ENIAC, the first
modern computer.
Meanwhile, over at the AT&T Bell Labs, in 1972 Dennis Ritchie was working
with two languages: B (for Bell) and BCPL (Basic Combined Programming
Language). Inspired by Pascal, Mr. Ritchie developed the C programming
language.
he built in 1822 that could be programmed to carry out different computations.
Move forward more than 100 years, where the U.S. government in
1942 used concepts from Babbage’s engine to create the ENIAC, the first
modern computer.
Meanwhile, over at the AT&T Bell Labs, in 1972 Dennis Ritchie was working
with two languages: B (for Bell) and BCPL (Basic Combined Programming
Language). Inspired by Pascal, Mr. Ritchie developed the C programming
language.
My 1st Program...
#include
#include
void main ()
{
clrscr ();
printf ("\n\n\n\n");
printf ("\t\t\t\"Life is Good...\"\n");
printf ("\t\t\t********************************");
getch ();
}
Next Step...
#include
#include
void main ()
{
clrscr ();
printf ("\n\n\n\n\n\n\n\n");
printf ("\t\t\t --------------------------- \n\n");
printf ("\t\t\t | IGCT, Info Computers, INDIA | \n\n");
printf ("\t\t\t --------------------------- ");
}