#include
#include
#define getx1y1
printf("Enter the order of Ist Matrix:");
scanf("%d%d",&x1,&y1);
#define getx2y2
printf("Enter the order of IIst Matrix:");
scanf("%d%d",&x2,&y2);
#define inputa
printf("Enter the value of a:");
for(i=0;i<=x1;i++)
for(j=0;j<=y1;j++)
scanf("%d",&a[i][j]);
#define inputb
printf("Enter the value of b:");
for(i=0;i<=x2;i++)
for(j=0;j<=y2;j++)
scanf("%d",&b[i][j]);
#define addab
for(i=0;i<=x1;i++)
{
for(j=0;j<=y1;j++)
{
c[i][j]=0;
for(k=0;k<=x1;k++)
{
c[i][j]=c[i][j]+a[i][k]*b[k][j];
}
}
}
#define output
printf("n The Result is:");
for(i=0;i<=x1;i++)
for(j=0;j<=y1;j++)
printf("tn%d",c[i][j]);
void main()
{
int a[5][5],b[5][5],c[5][5],i,j,k,x1,x2,y1,y2;
clrscr();
getx1y1;
getx2y2;
if((x1==x2)&&(y1==y2))
{
inputa;
inputb;
addab;
output;
}
else
printf("Matrix Addition Not Possible");
getch();
}
Program to show Macro Processor in C
Labels:
Basics of C,
c,
C Assignments
Program to create tower of Hanoi in C
yle: italic;">Definition : The Tower of Hanoi or Towers of Hanoi is a mathematical game or puzzle. It consists of three rods, and a number of disks of different sizes which can slide onto any rod. The puzzle starts with the disks in a neat stack in ascending order of size on one rod, the smallest at the top, thus making a conical shape.
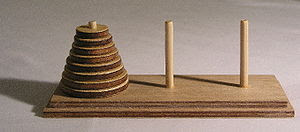
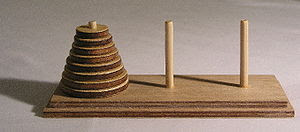
#include
void hanoi_tower(char,char,char,int);
void hanoi_tower(char peg1,char peg2,char pege3,int n)
{
if(n<=0) printf("\n Sorry Illegal Entry"); if(n==1) printf ("\n Move disk from %c to %c", pege1,pege3); else { hanoi_tower(peg1,peg3,peg2,n-1); hanoi_tower(peg1,peg2,peg3,1); hanoi_tower(peg2,peg1,peg3,n-1); } } Void main () { int n; printf("\n Input the number of disc:); scanf("%d", &n); printf("\n Tower of Hanoi for 5th DISC", n); hanoi_tower('x','y','z',n); }
Related Links :
Labels:
Basics of C,
C Assignments,
Projects In C
Round-robin CPU scheduling Program Algorithm in C
Definition : The term round-robin describes correspondence to a single address authored or signed by numerous individuals.
Output :
enter the process name : aaa
enter the processing time : 4
enter the process name : bbb
enter the processing time : 3
enter the process name : ccc
enter the processing time : 2
enter the process name : ddd
enter the processing time : 5
enter the process name : eee
enter the processing time : 1
OUTPUT :
p_name p_time w_time
aaa 4 9
bbb 3 3
ccc 2 6
ddd 5 10
eee 1 11
total waiting time : 39
average waiting time : 7.8000
Round-robin CPU scheduling Program Algorithm in C
#include
#include
main()
{
int st[10],bt[10],wt[10],tat[10],n,tq;
int i,count=0,pop=0,stat=0,xx,sq=0;
float awt=0.0,atat=0.0;
clrscr();
printf("Enter number of processes You Have:");
scanf("%d",&n);
printf("Enter burst time for sequences....:");
for(i=0;itq)
st[i]=st[i]-tq;
else
if(st[i]>=0)
{
xx=st[i];
st[i]=0;
}
sq=sq+xx;
tat[i]=sq;
}
if(n==count)
break;
}
for(i=0;i
Output :
enter the process name : aaa
enter the processing time : 4
enter the process name : bbb
enter the processing time : 3
enter the process name : ccc
enter the processing time : 2
enter the process name : ddd
enter the processing time : 5
enter the process name : eee
enter the processing time : 1
OUTPUT :
p_name p_time w_time
aaa 4 9
bbb 3 3
ccc 2 6
ddd 5 10
eee 1 11
total waiting time : 39
average waiting time : 7.8000
Related Links :
Program to convert Digit to words in C
Keywords : Program to convert Digit to words in C | how to convert Digit to words | Digit to words Conversion in C.
#include
#include
struct std{ int dig;
char wrd[20];
};
struct std
fig[]={{1,"one"},{2,"two"},{3,"three"},{4,"four"},{5,"five"},{6,"six"},
{7,"seven"},{8,"eight"},{9,"nine"},{10,"ten"},{11,"eleven"},{12,"twelve"},
{13,"thirteen"},
{14,"fourteen"},{15,"fifteen"},{16,"sixteen"},{17,"seventeen"},{18,"eighte
en"},{19,"nineteen"},
{20,"twenty"},{30,"thirty"},{40,"fourty"},{50,"fifty"},{60,"sixty"},{70,"s
eventy"},{80,"eighty"},
{90,"ninety"}};
int num1[5],i;
op0(long num)
{
for(i=0;i<20;i++)
if(fig[i].dig==num)
printf("%s",fig[i].wrd);
}
op1(long num)
{
//num1=num;
num1[0]=num/10;
num1[0]=num1[0]*10;
num1[1]=num%10;
for(i=0;i<27;i++)
{if(fig[i].dig==num1[0])
printf("%s ",fig[i].wrd);
}
for(i=0;i<20;i++)
{if(fig[i].dig==num1[1])
printf("%s",fig[i].wrd);
}
}
op2(long num)
{
int k,j=num%100;
k=num/100;
for(i=0;i<10;i++)
{if(fig[i].dig==k)
{printf("%s hundred ",fig[i].wrd);
if(j>0&&j<20)
op0(num%100);
else
op1(num%100);
}
}
}
op3(long num)
{
int k,j=num%1000;
k=num/1000;
for(i=0;i<10;i++)
{ if(fig[i].dig==k)
{ printf("%s thousand ",fig[i].wrd);
if(j>=1&&j<10)
op0(num%1000);
else if(j>10&&j<100)
op1(num%1000);
else
op2(num%1000);
}
}
}
op4(long num)
{
int k=num/1000,j;
j=num%1000;
if(k<=20)
{
op0(num/1000);
printf(" thousand ");
if(j>0&&j<10)
op0(num%1000);
if(j>10&&j<100)
op1(num%1000);
else op2(num%1000);
}
if((k>20)&&(k<100))
{
op1(num/1000);
printf(" thousand ");
if(j>0&&j<10)
op0(num%1000);
if(j>10&&j<100)
op1(num%1000);
else op2(num%1000);
}
//op2(num%1000);
}
op5(long num)
{
int k=num/100000;
int j=num%100000;
for(i=0;i<10;i++)
{ if(fig[i].dig==k)
{ printf("%s lakh ",fig[i].wrd);
if(j>0&&j<20)
op0(num%100000);
if(j>20&&j<100)
op1(num%100000);
if(j>100&&j<1000)
op2(num%100000);
if(j>1000&&j<10000)
op3(num%100000);
if(j>10000&&j<100000)
op4(num%100000);
}
}
}
main()
{
long num;
clrscr();
printf("Enter the number
");
scanf("%ld",&num);
if(num<=20)
op0(num);
if((num>20)&&(num/100==0))
op1(num);
if(num==100)
printf("hundred");
if(num>100&&num<1000)
op2(num);
if(num==1000) printf("one thousand");
if(num>1000&&num<10000)
op3(num);
if(num==10000) printf("ten thousand");
if(num>10000&&num<100000)
op4(num);
if(num==100000) printf("one lakh");
if(num>100000&&num<1000000)
op5(num);
if(num==1000000)
printf("One crore");
getch();
}
Related Links :
Labels:
Basics of C,
C Assignments
Ballon Fighter Game in C
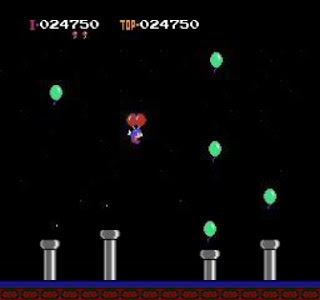
Program to create Ballon Fighter Game in C, a lovely game, nice to play...Enjoy.. :)
# include "graphics.h"
# include "conio.h"
# include "stdio.h"
# include "stdlib.h"
# include "dos.h"
#define ARROW_SIZE 7
#define BALLOON_SIZE 3
int flag_arrow=0,flag_balloon=1,count_arrow=6,count_balloon=10;
void *balloon,*bow,*arrow,*burst;
void *clear_balloon,*clear_burst;
void draw_balloon(int ,int );
void draw_burst ( int x, int y );
void draw_bow(int x,int y);
void draw_arrow(int x, int y);
void shoot(int *x, int *y);
int testkeys();
void fly(int *x, int *y);
void start();
void main()
{
int gmode = DETECT, gdriver , area ;
initgraph ( &gmode, &gdriver, "c: c gi" ) ;
setbkcolor(1);
start();
int maxx = getmaxx() ;
int maxy = getmaxy() ;
int
p=400,q=300,m=100,n=100,x=m,y=n,key,score=0,finish=0,level=1,l_flag=1;
char score1[5],ch,cnt_ball[5],char_level[2];
rectangle ( 0, 0, maxx, maxy - 10 ) ;
draw_burst(200,300);
area=imagesize(0,0,32,24);
burst=malloc(area);
getimage(200-16,300-12,200+16,300+12,burst);
putimage(200-16,300-12,burst,XOR_PUT);
draw_balloon(p,q);
area=imagesize(p-4*BALLOON_SIZE,q-5*BALLOON_SIZE,p+4*BALLOON_SIZE,q+7*BALL
OON_SIZE);
balloon=malloc(area);
getimage(p-4*BALLOON_SIZE,q-5*BALLOON_SIZE,p+4*BALLOON_SIZE,q+7*BALLOON_SI
ZE,balloon);
putimage(p-4*BALLOON_SIZE, q-5*BALLOON_SIZE, balloon, COPY_PUT);
draw_arrow(x ,y );
area = imagesize(x, y-ARROW_SIZE, x+(6*ARROW_SIZE), y+ARROW_SIZE);
arrow=malloc(area);
getimage(x, y-ARROW_SIZE, x+(6*ARROW_SIZE), y+ARROW_SIZE,arrow);
putimage(x, y-ARROW_SIZE,arrow,XOR_PUT);
draw_bow(x,y);
area=imagesize(x+25,y-65,x+66,y+65);
bow=malloc(area);
getimage(x+25,y-65,x+66,y+65,bow);
if ( balloon == NULL || burst == NULL || bow == NULL )
{
printf( "
Insufficient memory... Press any key " ) ;
getch() ;
closegraph() ;
restorecrtmode() ;
exit( 0 ) ;
}
while (!finish)
{
settextstyle(8,0,1);
setusercharsize(4,4,3,3);
outtextxy(getmaxx()/2-100,5,"LEVEL : ");
itoa(level,char_level,10);
setfillstyle(0,0);
bar(getmaxx()/2+40,15,getmaxx()/2+70,45);
outtextxy(getmaxx()/2+50,5,char_level);
rectangle(5,360,250,460);
if( flag_balloon && count_balloon>0 )
fly( &p, &q );
else
{
q = 400;
flag_balloon = 1;
}
if( kbhit() )
{
key = testkeys();
if(key==77)
flag_arrow = 1;
}
if( key == 27 )
break;
if (key == 80 &&!flag_arrow)
{
x=125;
putimage(x,y-65,bow,XOR_PUT);
if(y<300) key="0;" key ="=" x="125;">70)
y-=25;
putimage(x,y-65,bow,XOR_PUT);
draw_bow(x-25,y);
key=0;
}
if(count_arrow > 0 && count_balloon > 0)
{
if(score==100 && l_flag==1)
{
level=2;
count_balloon=8;
count_arrow=6;
l_flag=2;
}
if(score==180 && l_flag==2)
{
level=3;
count_balloon=6;
count_arrow=6;
l_flag=0;
}
if( key == 77 || flag_arrow)
{
shoot(&x,&y);
draw_bow(m,y);
if(x>(p-12) && x<(p+12) && y>(q-15) && y<(q+25)) { putimage(p-16,q-12,burst,COPY_PUT); sound(1500); delay(800); nosound(); putimage(p-16,q-12,burst,XOR_PUT); count_balloon--; settextstyle(10,0,1); setusercharsize(30,70,20,70); outtextxy(20,380,"Yippee! BALLOONS LEFT:"); setfillstyle(0,0); bar(200,370,230,400); itoa(count_balloon,cnt_ball,10); outtextxy(200,380,cnt_ball); flag_balloon=0; score+=20; itoa(score,score1,10); setfillstyle(0,0); bar(190,getmaxy()-50,230,getmaxy()-30); setcolor(RED); outtextxy(20,getmaxy()-50,"SCORE : "); outtextxy(190,getmaxy()-50,score1); } key=0; } } else { clearviewport(); setbkcolor(9); setcolor(10); settextstyle(4,0,7); setusercharsize(120,50,120,40); outtextxy(getmaxx()/2-220,getmaxy()/2-180,"GAME OVER"); settextstyle(8,0,1); setusercharsize(50,60,40,50); if(count_arrow<=0) outtextxy(getmaxx()/2-100,getmaxy()/2-70,"NO MORE ARROWS"); if(count_balloon<=0) outtextxy(getmaxx()/2-120,getmaxy()/2-70,"NO MORE BALLOONS"); outtextxy(getmaxx()/2-120,getmaxy()/2-20,"YOUR SCORE IS : "); itoa(score,score1,10); outtextxy(getmaxx()/2+150,getmaxy()/2-20,score1); if(level==1) outtextxy(getmaxx()/2-220,getmaxy()/2+20,"YOU REQUIRE TO PRACTICE MORE"); if(level==2) outtextxy(getmaxx()/2-70,getmaxy()/2+20,"WELL PLAYED"); if(level==3) outtextxy(getmaxx()/2-220,getmaxy()/2+20,"YOU ARE AN EFFICIENT SHOOTER"); outtextxy(getmaxx()/2-30,getmaxy()/2+50,"(Q)UIT"); settextstyle(1,0,1); setusercharsize(30,65,30,60); outtextxy(30,430,"THIS GAME HAS BEEN DEVELOPED BY RUCHIR BINDAL AND VINAY KUMAR GUPTA"); outtextxy(230,450,"THIRD YEAR, COMPUTER SCIENCE AND ENGINEERING"); while( getch() != 'q'); finish=1; break; } } free(bow); free(arrow); free(balloon); closegraph(); } void draw_balloon(int x,int y) { setcolor(RED); setfillstyle(1,RED); fillellipse(x,y,3*BALLOON_SIZE,4*BALLOON_SIZE); line(x,y+4*BALLOON_SIZE,x,y+6*BALLOON_SIZE); } void draw_burst ( int x, int y ) { setlinestyle(0,0,1); line ( x - 16, y - 12, x - 10, y - 2 ) ; line ( x - 10, y - 2, x - 16, y ) ; line ( x - 16, y, x - 10, y + 2 ) ; line ( x - 10, y + 2, x - 16, y + 12 ) ; line ( x - 16, y + 12, x - 6, y + 2 ) ; line ( x - 6, y + 2, x, y + 12 ) ; line ( x, y + 12, x + 6, y + 2 ) ; line ( x + 6, y + 2, x + 16, y + 12 ) ; line ( x - 16, y - 12, x - 6, y - 2 ) ; line ( x - 6, y - 2, x, y - 12 ) ; line ( x, y - 12, x + 6, y - 2 ) ; line ( x + 6, y - 2, x + 16, y - 12 ) ; line ( x + 16, y - 12, x + 10, y - 2 ) ; line ( x + 10, y - 2, x + 16, y ) ; line ( x + 16, y, x + 10, y + 2 ) ; line ( x + 10, y + 2, x + 16, y + 12 ) ; } void draw_bow(int x,int y) { setcolor(RED); setlinestyle(0,0,1); line(x+32,y-49,x+32,y+49); setlinestyle(0,0,3); arc(x,y,300,60,60); arc(x+34,y-56,100,220,6); arc(x+34,y+56,140,260,6); } void shoot(int *x, int *y) { char cnt_arrow[5]; putimage(*x, *y-ARROW_SIZE, arrow, COPY_PUT); delay(3); putimage(*x, *y-ARROW_SIZE, arrow, XOR_PUT); *x+=ARROW_SIZE; if (*x>590)
{
*x=155;
flag_arrow=0;
count_arrow--;
settextstyle(10,0,1);
setusercharsize(30,70,20,70);
outtextxy(20,400,"ARROWS LEFT :");
setfillstyle(0,WHITE);
bar(200,400,220,425);
itoa(count_arrow,cnt_arrow,10);
outtextxy(200,400,cnt_arrow);
}
}
void draw_arrow(int x, int y)
{
setlinestyle(0,0,2);
moveto(x, y);
linerel(6*ARROW_SIZE, 0);
linerel(-2*ARROW_SIZE, -1*ARROW_SIZE+1);
linerel(0, 2*ARROW_SIZE-1);
linerel(2*ARROW_SIZE, -1*ARROW_SIZE);
}
int testkeys()
{
union REGS ii, oo ;
ii.h.ah = 0 ;
int86 ( 22, &ii, &oo ) ;
/* if ascii code is not 0 */
if ( oo.h.al )
return ( oo.h.al ) ; /* return ascii code */
else
return ( oo.h.ah ) ; /* return scan code */
}
void fly(int *x, int *y)
{
int x1;
putimage(*x-4*BALLOON_SIZE, *y-5*BALLOON_SIZE, balloon, COPY_PUT);
delay(20);
char cnt_ball[5];
putimage(*x-4*BALLOON_SIZE, *y-5*BALLOON_SIZE, balloon, XOR_PUT);
*y-=BALLOON_SIZE;
if(*y<= 20) { *y=400; x1=450+rand()%150; *x=x1; count_balloon--; settextstyle(10,0,1); setusercharsize(30,70,20,70); outtextxy(20,380,"BALLOONS LEFT:"); setfillstyle(0,0); bar(200,370,230,400); itoa(count_balloon,cnt_ball,10); outtextxy(200,380,cnt_ball); } } void start() { setbkcolor(0); settextstyle(7,0,0); outtextxy(10,400," PRESS ANY KEY TO CONTINUE...."); settextstyle(1,0,0); setcolor(4); setusercharsize(25,15,20,4); outtextxy(85,120,"BALLOON SHOOTING"); float octave[7] = { 130.81, 146.83, 164.81, 174.61, 196, 220, 246.94 }; while( !kbhit() ) { sound( octave[ random(7) ]*4 ); delay(300); } nosound(); getch(); clearviewport(); rectangle(1,1,638,478); settextstyle(3,0,1); setusercharsize(50,30,50,30); outtextxy(150,10,"INSTRUCTIONS"); setbkcolor(10); settextstyle(1,0,1); setusercharsize(40,70,20,20); outtextxy(10,70,"1. You can play three levels."); outtextxy(10,110,"2. You can move the bow UP and DOWN with the help of arrow keys."); outtextxy(10,150,"3. Press right arrow key to shoot the arrow."); outtextxy(10,190,"4. You score 20 points every time you shoot the balloon."); outtextxy(10,230,"5. First level has 6 arrows and 10 balloons."); outtextxy(10,270,"6. You require to score 100 points to enter the second level."); outtextxy(10,310,"7. Second level has 6 arrows and 8 balloons."); outtextxy(10,350,"8. You require to score 200 points to enter the third level."); outtextxy(10,390,"9. Third level has 6 arrows and 6 balloons."); settextstyle(7,0,1); outtextxy(150,430,"PRESS ANY KEY TO CONITINUE"); getch(); setusercharsize(1,1,1,1); settextstyle(0,0,0); setbkcolor(10); clearviewport(); }
Related Links :
Labels:
C Assignments,
Graphics In C,
Projects In C
Beautiful Animation In C
Program to create Beautiful Animation In C.
#include
#include
#include
#include
void main()
{
int d=2,k=0;
int gd=DETECT,gm;
initgraph(&gd,&gm,"c:\tc\bgi");
setcolor(2);
outtextxy(300,430,"Please Press any key to continue....");
setcolor(5);
setwritemode(1);
rectangle(180,120,380,320);
getch();
clearviewport();
while(!kbhit())
{
d%=91;
setcolor(k);
rectangle(180+d,120+d,380-d,320-d);
delay(40);
d+=2;
k++;
}
getch();
}
Related Links :
Labels:
Basics of C,
C++ Assignment,
Graphics In
Folder Lock Software || Folder Protection Software Code in C || Folder Protection Software for XP, Windows Vista & Windows 7
#include
#include
#include
void main()
{
FILE *p;
char ch,s[100];
char r[100]="REN ";
char u[]=".{21EC2020-3AEA-1069-A2DD-08002B30309D} ";
char v[50];
int choice,i;
clrscr();
p=fopen("C:\pop.bat","w+");
if(p==NULL)
{
printf("
Error in opening the file a.c
");
exit(0);
}
printf("This software can convert your File/Folder to Control Panel Format
and can be Restore again.");
printf(" Enter the path of the file you Want to Lock");
fflush(stdin);
gets(s);
for(i=0;i<25;i++)
fputs("echo This software is not responsible for any loss in data",p);
printf("Enter choice :");
printf("1.Protect Folder/File");
printf("2.Unprotect folder/File");
printf("3.Exit");
scanf("%d",&choice);
switch(choice)
{
case 1:
strcat(r,s);
printf("Enter new name of your folder/file");
fflush(stdin);
gets(v);
strcat(r," ");
strcat(r,v);
strcat(r,u);
break;
case 2:
strcat(r,s);
strcat(r,u);
printf("
Enter new name of your folder/file
");
fflush(stdin);
gets(v);
strcat(r,v);
break;
default:
fclose(p);
remove("C:\pop.bat");
exit(0);
}
{
fputs(r,p);
for(i=0;i<25;i++)
fputs("echo This software is not responsible for any loss in data ",p);
fputs("exit",p);
}
fclose(p);
system("C:\pop.bat");
remove("C:\pop.bat");
}
Related Links :
Labels:
Basics of C,
c,
C Assignments
String len
#include
#include
#include
#define PACKAGE "strl"
#define VERSION "1.0.0"
void print_help(int exval);
int main(int argc, char *argv[]) {
FILE *fp = stdin;
char line[1024];
int opt = 0;
int strip_newline = 0;
while((opt = getopt(argc, argv, "hvs")) != -1) {
switch(opt) {
case 'h':
print_help(0);
break;
case 'v':
fprintf(stdout, "%s %s\n", PACKAGE, VERSION);
exit(0);
break;
case 's':
strip_newline = 1;
break;
case '?':
fprintf(stderr, "%s: Error - No such option: `%c'\n\n", PACKAGE, optopt);
print_help(1);
break;
} /* switch */
} /* while */
if((argc - optind) == 0) {
while((fgets(line, 1024, fp)) != NULL) {
if(strip_newline == 1)
if(line[strlen(line) - 1] == '\n')
line[strlen(line) - 1] = '\0';
printf("%4d %s\n", strlen(line), line);
} /* while */
} else {
for(; optind < argc; optind++) {
if(freopen(argv[optind], "r", fp) == NULL) {
perror(argv[optind]);
continue;
}
while((fgets(line, 1024, fp)) != NULL) {
if(strip_newline == 1)
if(line[strlen(line) - 1] == '\n')
line[strlen(line) - 1] = '\0';
printf("%4d %s\n", strlen(line), line);
} /* while */
} /* for */
} /* else */
return 0;
}
void print_help(int exval) {
printf("%s,%s compute string length\n", PACKAGE, VERSION);
printf("Usage: %s [-h] [-v] [-s] FILE1 FILE2 ...\n\n", PACKAGE);
printf("Options:\n");
printf(" -h print this help and exit\n");
printf(" -v print version and exit\n");
printf(" -s strip trailing newlines\n\n");
printf(" If no filenames given, strings\n");
printf(" are read from stdin().\n\n");
printf(" This program does basicly the same as\n");
printf(" `${#varname}' in the GNU/Linux bash shell.\n\n");
exit(exval);
}
Related Links :
Labels:
Basics of C,
C Assignments,
Projects In C
Dedekind ETA function
#include
#include
#include
#include
#define PACKAGE "eta"
#define VERSION "0.0.1"
#define MAXLINE 1024
/* Defination : The Dedekind eta function, named after Richard Dedekind, is a function defined on the upper half-plane of complex numbers, where the imaginary part is positive. */
void print_help(int exval);
/* print program version and exit with exval */
void print_version(int exval);
/* `\056' `float' detection ? */
/* returns -1 on error, 1 on `no dot', 0 on `dot' */
int is_float(char *val);
int main(int argc, char *argv[]) {
char line[MAXLINE]; /* fgets buff */
int overview_flag = 0; /* print overview only */
int count = 0; /* nr of input elements */
float total = 0; /* eta */
float num = 0; /* the actual element */
int opt = 0; /* parsed opt nr */
float highest = 0; /* the lowest encounterd nr */
float lowest = 0; /* the highest encounterd nr */
/* option parser */
while((opt = getopt(argc, argv, "hve")) != -1) {
switch(opt) {
case 'h': /* print help and exit */
print_help(0);
case 'v': /* print version and exit */
print_version(0);
case 'e':
overview_flag = 1;
break;
case '?':
fprintf(stderr, "%s: Error - No such option: `%c'\n\n", PACKAGE, optopt);
print_help(1);
}
}
/* no remaining argumenst left ? read stdin() */
if((argc - optind) == 0) {
while((fgets(line, MAXLINE, stdin)) != NULL) {
/* strip newlines */
if(line[strlen(line) - 1] == '\n')
line[strlen(line) - 1] = '\0';
count++;
if(is_float(line) == 0)
num = atof(line);
else
num = atoi(line);
if(count == 1) highest = num, lowest = num;
if(num > highest) highest = num;
if(num < lowest =" num;" num =" atoi(argv[optind]);" num =" atof(argv[optind]);" count ="="" highest =" num," lowest =" num;"> highest) highest = num;
if(num < lowest =" num;" overview_flag ="="" retval =" -1;" retval =" 1;" retval =" 0;">
Related Links :
Labels:
Basics of C,
C Assignments,
Projects In C
program to print the pyramid of numbers.
program to print the pyramid of numbers as follows :
1
123
12345
1234567
123456789
1
123
12345
1234567
123456789
void prntsp(int n)
{
int i;
for(i = 0; i<=n; i++)
printf(" "); // printing n spaces
}
void pyraprint()
{
int i, j, sp = 4;
for(i = 1; i<= 5; i++)
{
prntsp(sp);
for(j = 1; j<= 2*i-1; j++)
{
printf("%d",j);
}
prntsp(sp);
sp--;
printf("\n");
}
}
int main()
{
pyraprint();
return 0;
}
Related Links :
Labels:
Basics of C,
C Assignments
Program to factorize the given number.
Program to factorize the given number.
int main()
{
int pos_1, pos_2;
char ch;
char input[100];
gets(input); // reading the String
pos_1 = 0; // first position
pos_2 = strlen(input) - 1; // last position
while(pos_1 < pos_2)
{
ch = input[pos_1];
input[pos_1] = input[pos_2];
input[pos_2] = ch;
pos_1++;
pos_2--;
}
printf("%s\n",input);
return 0;
}
Related Links :
Labels:
Basics of C,
C Assignments
A program to print the following pyramid.
A
AB
ABC
ABCD
ABCDE
ABCDEF
AB
ABC
ABCD
ABCDE
ABCDEF
void Print()
{
int i, j;
for(i = 0; i<6; i++)
{
for(j = 0; j<= i; j++)
{
printf("%c",j+'A');
}
printf("\n");
}
}
int main()
{
Print();
return 0;
}
Related Links :
Labels:
Basics of C,
C Assignments
Program to print all paindromic number from 1 to n.
int isPalindrm(int n)
{
char str[100];
sprintf(str,"%d",n);
strrev(str);
int m = atoi(str); // converting the reversed string to an integer
return n == m; // comparing reversed number with orginal number, retrun 1 when true, 0 otherwise
}
void printPalin(int n)
{
int i;
for(i = 1; i<=n; i++)
{
if(isPalindrm(i)) printf("%d ",i);
}
}
int main()
{
int n;
scanf("%d",&n);
printPalin(n);
return 0;
}
Related Links :
Labels:
Basics of C,
C Assignments
Program to find LCM (Lowest Common multiple) of given two numbers in C
This Program is used to find LCM (Lowest Common multiple) of given two numbers in C langauge.
int GCD(int n, int m)
{
int temp;
while( m > 0)
{
temp = n;
n = m;
m = temp % m;
}
return n;
}
int LCM(int n, int m) {
int product = n*m;
int gcd = GCD(n,m);
int lcm = product/gcd;
return lcm;
}
int main()
{
int n, m;
scanf("%d%d",&n,&m);
printf("%d\n",LCM(n,m));
return 0;
}
Related Links :
Labels:
Basics of C,
C Assignments
Program to find Greatest Common Divisor (GCD) of given two integers.
int DivisorN[100];
int DivisorM[100];
int index1, index2;
int N, M;
void findDivisorOfN()
{
int i;
for(i = 1; i<=N/2; i++)
{
if(N%i == 0) {
DivisorN[index1++] = i;
}
}
DivisorN[index1++] = N;
}
void findDivisorOfM()
{
int i;
for(i = 1; i<=M/2; i++)
{
if(M%i == 0) {
DivisorM[index2++] = i;
}
}
DivisorM[index2++] = M;
}
int findGreatestCommonDivisor()
{ // Find the height Common divisor between N and M
int i, j;
for(i = index1-1; i>=0; i--)
{
for(j = index2-1; j>=0; j--)
{
if(DivisorN[i] == DivisorM[j])
{
return DivisorN[i];
}
}
}
}
int main()
{
scanf("%d %d",&N,&M);
findDivisorOfN();
findDivisorOfM();
printf("%d\n",findGreatestCommonDivisor());
return 0;
}
Related Links :
Labels:
Basics of C,
C Assignments
Program to convert a decimal number to binary number.
void Binary(int n)
{
int digit[100], int index = 0;
while(n)
{
n!= 0 or n > 0
digit[index++] = n%2;
n /= 2;
}
for(int i = index-1; i>=0; i--)
{
printf("%d",digit[i]);
}
}
int main()
{
int n;
scanf("%d",&n);
Binary(n);
return 0;
}
Related Links :
Labels:
C Assignments
program which inpute an integer and print equivalent hexadecimial value.
main()
{
int n;
printf("Enter Ani number..");
scanf("%d",&n);
printf("%x\n",n);
}
{
int n;
printf("Enter Ani number..");
scanf("%d",&n);
printf("%x\n",n);
}
Related Links :
Labels:
Basics of C
what is Debugging with GNU Debugger (GDB)
The debugger is the program that you use to figure out why your program isn’t behaving the way you think it should.You’ll be doing this a lot. The GNU Debugger (GDB) is the debugger used by most Linux programmers.You can use GDB to step through your code, set breakpoints, and examine the value of local variables.
Related Links :
C header files in unix & its Working
You can learn a lot about the system functions that are available and how to use them by looking at the system header files.These reside in /usr/include and /usr/include/sys. If you are getting compile errors from using a system call, for instance, take a look in the corresponding header file to verify that the function’s signature is the same as what’s listed in the man page.
On Linux systems, a lot of the nitty-gritty details of how the system calls work are reflected in header files in the directories /usr/include/bits, /usr/include/asm, and /usr/include/linux. For instance, the numerical values of signals are defined in /usr/include/bits/signum.h.
These header files make good reading for inquiring minds. Don’t include them directly in your programs, though; always use the header files in /usr/include or as mentioned in the man page for the function you’re using.
On Linux systems, a lot of the nitty-gritty details of how the system calls work are reflected in header files in the directories /usr/include/bits, /usr/include/asm, and /usr/include/linux. For instance, the numerical values of signals are defined in /usr/include/bits/signum.h.
These header files make good reading for inquiring minds. Don’t include them directly in your programs, though; always use the header files in /usr/include or as mentioned in the man page for the function you’re using.
Related Links :
Program to Visual Bubble Sort in C
void object(int,int,int);
void bubble(int);
void flow(int,int);
void mixing(int,int);
int el[10];
void main()
{
int n,i,d=0,m=0;
clrscr();
printf("Enter the No of Elements : " );
scanf("%d",&n);
for(i=0;i
{
printf("Enter the %d Element : ",i+1);
scanf("%d",&el[i]);
}
initgraph(&d,&m,"");
settextstyle(3,0,1);
outtextxy(250,50,"GIVEN NUMBERS ");
// outtextxy(250,260," AFTER SORTING ");
// line(0,getmaxy()/2,getmaxx(),getmaxy()/2);
for(i=0;i
{
object(100+i*50,150,el[i]);
getch();
}
bubble(n);
/* for(i=0;i
{
object(100+i*50,350,el[i]);
getch();
}*/
getch();
}
void bubble(int n)
{
int i,j,temp;
for(i=0;i
for(j=i;j
if(el[i]>el[j+1])
{
flow(i,j+1);
temp=el[i];
el[i]=el[j+1];
el[j+1]=temp;
}
}
void object(int x,int y,int no)
{
char s[8];
sprintf(s,"%d",no);
circle(x,y,15);
settextstyle(2,0,6);
outtextxy(x-3,y-10,s);
}
void flow(int f,int s)
{
int i;
for(i=0;i<50;i++)
{
setcolor(WHITE);
object(100+f*50,150+i*4,el[f]);
delay(15);
setcolor(0);
object(100+f*50,150+i*4,el[f]);
}
setcolor(WHITE);
object(100+f*50,150+i*4,el[f]);
for(i=0;i<50;i++)
{
setcolor(WHITE);
object(100+s*50,150+i*4,el[s]);
delay(10);
setcolor(0);
object(100+s*50,150+i*4,el[s]);
}
setcolor(WHITE);
object(100+s*50,150+i*4,el[s]);
mixing(f,s);
for(i=50;i>0;i--)
{
setcolor(WHITE);
object(100+f*50,150+i*4,el[s]);
delay(10);
setcolor(0);
object(100+f*50,150+i*4,el[s]);
}
setcolor(WHITE);
object(100+f*50,150+i*4,el[s]);
for(i=50;i>0;i--)
{
setcolor(WHITE);
object(100+s*50,150+i*4,el[f]);
delay(15);
setcolor(0);
object(100+s*50,150+i*4,el[f]);
}
setcolor(WHITE);
object(100+s*50,150+i*4,el[f]);
}
void mixing(int f,int s)
{
int i;
for(i=0;i<(s-f)*50;i++)
{
setcolor(WHITE);
object(100+f*50+i,350,el[f]);
object(100+s*50-i,350,el[s]);
delay(20);
setcolor(0);
object(100+f*50+i,350,el[f]);
object(100+s*50-i,350,el[s]);
}
setcolor(WHITE);
object(100+f*50+i,350,el[f]);
object(100+s*50-i,350,el[s]);
}
Related Links :
Labels:
Basics of C,
C Assignments
Prpgram to reverse the given number & find Sum of it
#include "stdio.h"
#include "math.h"
int main()
{
int number, number2;
char str[100];
printf("Enter Any Number..");
scanf("%d",&number);
sprintf(str,"%d",number);
strrev(str);
number2 = atoi(str);
printf("%d\n",number+number2);
return 0;
}
Related Links :
Labels:
Basics of C,
C Assignments
Program to print the ascii equivalent values of all chararters in the given string
int ascii_value(char c);
void main()
{
int i,a;
char c;
clrscr();
printf("Please enter any string...");
printf("String will be terminated if you pressing Ctrl-Z.");
printf("Enter String: ");
for (i=0;(c=getchar())!=EOF;i++)
{
a=ascii_value(c);
printf("%d%c",a,' ');
}
printf("are the ascii values of the characters of the given string");
getch();
}
int ascii_value(char c)
{
int a;
a=(int)c;
return(a);
}
Related Links :
Labels:
Basics of C,
C Assignments
Program to find the type of the triangle made by input or given values of its coordinates of its vertices in c.
Program to find the type of the triangle made by input or given values of its coordinates of its vertices in c.
#include
#include
#include
#include
void main()
{
float x1,y1,x2,y2,x3,y3;
float a,b,c,m1,m2,m3;
clrscr();
printf("Enter coordinates of vertex A(x & y respectively)... ");
scanf("%f%f",&x1,&y1);
printf("Enter coordinates of vertex B(x & y respectively)... ");
scanf("%f%f",&x2,&y2);
printf("Enter coordinates of vertex C(x & y respectively)... ");
scanf("%f%f",&x3,&y3);
if ((x1==x2 && x2==x3)||(y1==y2 && y2==y3))
{
printf("Sorry ..These coordinates can't represent any triangle.");
printf("A,B & C are colinear & thus consitute a line.");
getch();
exit(0);
}
else
{
m1=(y2-y1)/(x2-x1);
m2=(y3-y2)/(x3-x2);
m3=(y3-y1)/(x3-x1);
}
if (m1==m2||m2==m3||m3==m1)
{
printf("These coordinates can't represent a triangle.");
printf("A,B & C are colinear & thus consitute a line.");
printf("Thanks Good Day! BYE.");
getch();
exit(0);
}
a = sqrt(pow((x2-x3),2) + pow((y2-y3),2));
b = sqrt(pow((x3-x1),2) + pow((y3-y1),2));
c = sqrt(pow((x2-x1),2) + pow((y2-y1),2));
printf("Length of side AB is = %f",c);
printf("Length of side BC is = %f",a);
printf("Length of side CA is = %f",b);
if (a==b==c)
printf("Triangle made by these vertices is an Equilateral Triangle.");
else if (a==b||b==c||c==a)
{
if (a==b==c);
else
printf("Triangle made by these vertices is an Isosceles Triangle.");
}
else if (a!=b && b!=c && c!=a)
printf("Triangle made by these vertices is a scalene triangle.");
getch();
}
Related Links :
Labels:
Basics of C,
C Assignments
Prpgram to count vowels in given string by using vfork method (use of Vfork Function in c)
#include "stdio.h"
#include "dirent.h"
int main()
{
int j,n,a,i,e,o,u;
char str[50];
a=e=i=o=u=0;
pid_t pid;
if((pid=vfork())<0)
{
perror("FORK ERROR");
exit(1);
}
if(pid==0)
{
printf("Enter the String to count vowels:");
gets(str);
_exit(1);
}
else
{
n=strlen(str);
for(j=0;j
{
if(str[j]=='a' || str[j]=='A')
a++;
else if(str[j]=='e' || str[j]=='E')
e++;
else if(str[j]=='i' || str[j]=='I')
i++;
else if(str[j]=='o' || str[j]=='O')
o++;
else if(str[j]=='u' || str[j]=='U')
u++;
}
printf("Vowels Counting");
printf("Number of A : %d",a);
printf("Number of E : %d",e);
printf("Number of I : %d",i);
printf("Number of O : %d",o);
printf("Number of U : %d",u);
printf("Total vowels : %d",a+e+i+o+u);
exit(1);
}
}
Related Links :
Labels:
Unix Linux Based Programs
Subscribe to:
Posts (Atom)
If you face any Problem in viewing code such as Incomplete "For Loops" or "Incorrect greater than or smaller" than equal to signs then please collect from My Web Site CLICK HERE
More Useful Topics... |
|
History Of C..
In the beginning was Charles Babbage and his Analytical Engine, a machine
he built in 1822 that could be programmed to carry out different computations.
Move forward more than 100 years, where the U.S. government in
1942 used concepts from Babbage’s engine to create the ENIAC, the first
modern computer.
Meanwhile, over at the AT&T Bell Labs, in 1972 Dennis Ritchie was working
with two languages: B (for Bell) and BCPL (Basic Combined Programming
Language). Inspired by Pascal, Mr. Ritchie developed the C programming
language.
he built in 1822 that could be programmed to carry out different computations.
Move forward more than 100 years, where the U.S. government in
1942 used concepts from Babbage’s engine to create the ENIAC, the first
modern computer.
Meanwhile, over at the AT&T Bell Labs, in 1972 Dennis Ritchie was working
with two languages: B (for Bell) and BCPL (Basic Combined Programming
Language). Inspired by Pascal, Mr. Ritchie developed the C programming
language.
My 1st Program...
#include
#include
void main ()
{
clrscr ();
printf ("\n\n\n\n");
printf ("\t\t\t\"Life is Good...\"\n");
printf ("\t\t\t********************************");
getch ();
}
Next Step...
#include
#include
void main ()
{
clrscr ();
printf ("\n\n\n\n\n\n\n\n");
printf ("\t\t\t --------------------------- \n\n");
printf ("\t\t\t | IGCT, Info Computers, INDIA | \n\n");
printf ("\t\t\t --------------------------- ");
}